请你分别为我写出这四种线性结构的代码示例,要求使用C语言编写
时间: 2024-09-09 16:11:39 浏览: 38
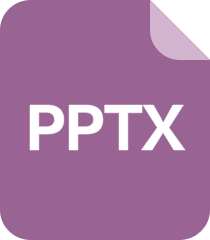
DataStructure C语言版 数据结构与算法教程PPT课件 格式化输入输出 共27页.pptx
四种常见的线性结构包括:数组、链表、栈和队列。以下是使用C语言实现这四种数据结构的基本示例代码。
1. 数组(Array):
```c
#include <stdio.h>
#define SIZE 5
int main() {
int arr[SIZE] = {1, 2, 3, 4, 5}; // 声明并初始化一个整型数组
int i;
// 打印数组元素
for (i = 0; i < SIZE; i++) {
printf("arr[%d] = %d\n", i, arr[i]);
}
return 0;
}
```
2. 链表(Linked List):
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建链表节点
Node* createNode(int data) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (newNode == NULL) {
exit(1); // 分配内存失败,退出程序
}
newNode->data = data;
newNode->next = NULL;
return newNode;
}
int main() {
Node* head = createNode(1); // 创建链表头节点
head->next = createNode(2);
head->next->next = createNode(3);
// 打印链表元素
Node* current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
// 释放链表内存
current = head;
while (current != NULL) {
Node* temp = current;
current = current->next;
free(temp);
}
return 0;
}
```
3. 栈(Stack):
```c
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 10
typedef struct Stack {
int data[MAXSIZE];
int top;
} Stack;
// 初始化栈
void initStack(Stack* s) {
s-1;
}
// 判断栈是否为空
int isEmpty(Stack* s) {
return s->top == -1;
}
// 判断栈是否已满
int isFull(Stack* s) {
return s->top == MAXSIZE - 1;
}
// 入栈操作
void push(Stack* s, int value) {
if (isFull(s)) {
printf("Stack is full!\n");
} else {
s->data[++s->top] = value;
}
}
// 出栈操作
int pop(Stack* s) {
if (isEmpty(s)) {
printf("Stack is empty!\n");
return -1; // 假设栈中不包含-1,可以作为错误返回值
} else {
return s->data[s->top--];
}
}
int main() {
Stack s;
initStack(&s);
push(&s, 1);
push(&s, 2);
push(&s, 3);
printf("%d\n", pop(&s));
printf("%d\n", pop(&s));
printf("%d\n", pop(&s));
return 0;
}
```
4. 队列(Queue):
```c
#include <stdio.h>
#include <stdlib.h>
#define MAXSIZE 5
typedef struct Queue {
int data[MAXSIZE];
int front;
int rear;
} Queue;
// 初始化队列
void initQueue(Queue* q) {
q->front = q->rear = 0;
}
// 判断队列是否为空
int isEmpty(Queue* q) {
return q->front == q->rear;
}
// 判断队列是否已满
int isFull(Queue* q) {
return (q->rear + 1) % MAXSIZE == q->front;
}
// 入队操作
void enqueue(Queue* q, int value) {
if (isFull(q)) {
printf("Queue is full!\n");
} else {
q->data[q->rear] = value;
q->rear = (q->rear + 1) % MAXSIZE;
}
}
// 出队操作
int dequeue(Queue* q) {
if (isEmpty(q)) {
printf("Queue is empty!\n");
return -1; // 假设队列中不包含-1,可以作为错误返回值
} else {
int value = q->data[q->front];
q->front = (q->front + 1) % MAXSIZE;
return value;
}
}
int main() {
Queue q;
initQueue(&q);
enqueue(&q, 1);
enqueue(&q, 2);
enqueue(&q, 3);
printf("%d\n", dequeue(&q));
printf("%d\n", dequeue(&q));
printf("%d\n", dequeue(&q));
return 0;
}
```
阅读全文
相关推荐
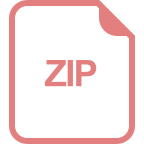
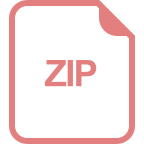
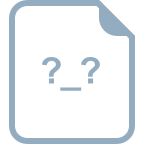
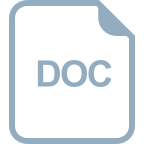
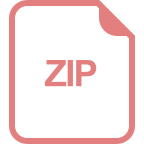
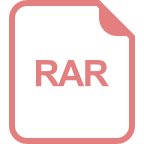
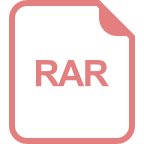
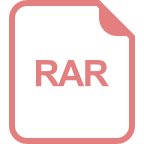
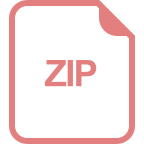
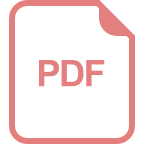
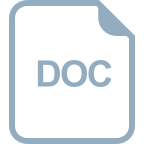
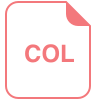
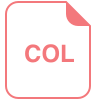




