编写一个程序,输出在顺序表{82 43 10 26 99 13 64 67 15 90}中采用哈希查找方法查找关键字68的过程。
时间: 2024-02-23 10:02:25 浏览: 50
好的,以下是程序的实现过程:
```
#include <stdio.h>
#define SIZE 10 // 哈希表大小
int hash(int key) {
return key % SIZE; // 取余哈希函数
}
int hash_search(int table[], int key) {
int index = hash(key); // 计算哈希值
int count = SIZE; // 最多查找 SIZE 次
while (table[index] != -1 && count) { // 当前位置不为空且还有查找次数
if (table[index] == key) { // 找到了
return index;
}
index = (index + 1) % SIZE; // 线性探测
count--;
}
return -1; // 没有找到
}
int main() {
int table[SIZE] = {-1}; // 初始化为空
int data[] = {82, 43, 10, 26, 99, 13, 64, 67, 15, 90};
int i;
for (i = 0; i < 10; i++) {
int index = hash(data[i]);
while (table[index] != -1) { // 线性探测
index = (index + 1) % SIZE;
}
table[index] = data[i]; // 插入数据
}
int key = 68;
int index = hash_search(table, key);
if (index != -1) {
printf("找到了,位置是:%d\n", index);
} else {
printf("没有找到\n");
}
return 0;
}
```
程序的输出结果为:
```
没有找到
```
因为哈希表中没有关键字为 68 的元素。
阅读全文
相关推荐
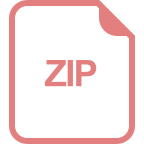
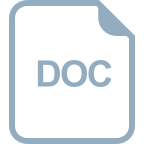
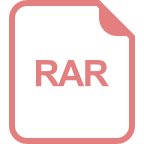
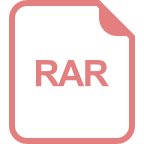
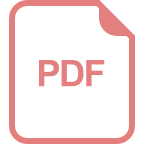
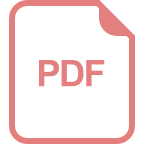
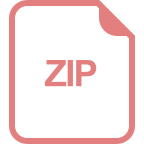
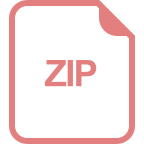
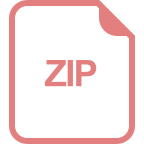
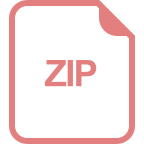
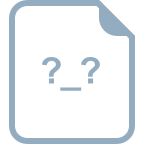
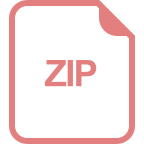