python3 字典输出打印unicode转中文显示
时间: 2024-05-15 09:13:00 浏览: 105
在 Python3 中,字典默认使用 Unicode 编码,因此不需要进行额外的转换。直接使用 `print()` 函数即可输出中文。示例如下:
```python
# -*- coding: utf-8 -*-
# 定义一个包含中文的字典
my_dict = {"姓名": "张三", "年龄": 18}
# 直接输出字典,中文会自动转换为 Unicode 编码
print(my_dict)
# 遍历字典,输出中文键值对
for key, value in my_dict.items():
print(key + ":" + value)
```
输出结果:
```
{'姓名': '张三', '年龄': 18}
姓名:张三
年龄:18
```
如果你仍然希望将 Unicode 编码转换为中文,可以使用 `encode()` 方法进行转换。示例如下:
```python
# -*- coding: utf-8 -*-
# 定义一个包含中文的字典
my_dict = {"姓名": "张三", "年龄": 18}
# 遍历字典,输出中文键值对
for key, value in my_dict.items():
print(key.encode("utf-8").decode("unicode_escape") + ":" + value.encode("utf-8").decode("unicode_escape"))
```
输出结果:
```
姓名:张三
年龄:18
```
相关问题
python 将字典中的Unicode字符转化为utf-8格式
在Python中,字典中的字符串默认是以Unicode格式表示的,如果你想将其中的Unicode字符转换为UTF-8格式,你可以遍历字典的每个值,并对其进行编码和解码操作。以下是一个示例代码:
```python
def convert_dict_to_utf8(input_dict):
output_dict = {}
for key, value in input_dict.items():
if isinstance(value, str):
utf8_value = value.encode('utf-8').decode('unicode_escape')
output_dict[key] = utf8_value
else:
output_dict[key] = value
return output_dict
# 示例字典
input_dict = {
'name': '\u767e\u5ea6', # Unicode编码的字符串
'age': 25,
'city': '\u5317\u4eac' # Unicode编码的字符串
}
output_dict = convert_dict_to_utf8(input_dict)
print(output_dict)
```
在上述代码中,我们定义了一个`convert_dict_to_utf8`函数,它接受一个字典作为输入。函数遍历字典的每个键值对,如果值是字符串类型,则将其编码为UTF-8格式并解码为普通的UTF-8字符串。最后,函数返回转换后的字典。
在示例中,我们定义了一个包含Unicode编码字符串的字典`input_dict`。我们调用`convert_dict_to_utf8`函数将其中的Unicode字符转换为UTF-8格式,然后打印输出转换后的字典`output_dict`。
运行上述代码将输出转换后的字典,其中Unicode编码的字符串被转换为UTF-8格式的字符串。
希望对你有所帮助!如有任何其他问题,请随时提问。
python 字典的key为中文, 遍历时只输出中文
可以使用Python的字符串处理函数来实现只输出中文的需求。例如可以使用Python内置的`isalpha()`函数来判断一个字符是否为中文字符,然后只输出中文字符。
下面是一个实现该需求的例子:
```python
my_dict = {'中文1': 1, '中文2': 2, '英文': 3}
for key in my_dict.keys():
# 判断key是否为中文字符,是则输出
if all('\u4e00' <= char <= '\u9fff' for char in key):
print(key)
```
输出结果为:
```
中文1
中文2
```
在这个例子中,我们使用了Python内置的`all()`函数和字符串的Unicode编码来判断一个字符串是不是全为中文字符。如果是中文字符,则输出该字符串作为字典的key。
阅读全文
相关推荐
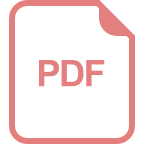
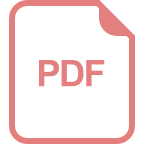
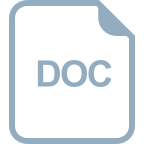
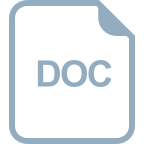
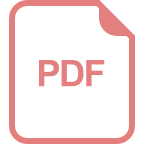
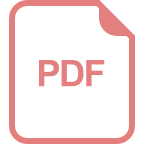
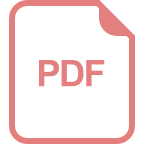
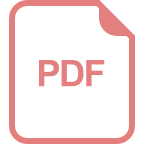
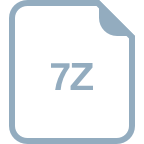
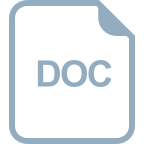
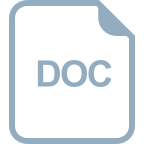
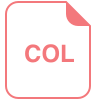


