vue3加arco design vue组件 如何使用a-upload实现图片上传功能
时间: 2023-11-22 08:53:34 浏览: 839
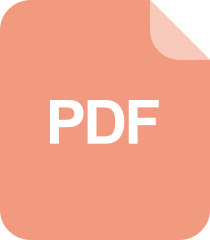
vue项目实现图片上传功能
要使用 arco design 的 a-upload 组件实现图片上传功能,你需要先安装 arco design 和 axios(用于发送上传请求)两个依赖。
1. 安装 arco design 和 axios
```bash
npm install arco-design axios --save
```
2. 导入 a-upload 组件和 axios
```vue
<template>
<div>
<a-upload
:action="uploadUrl"
:beforeUpload="beforeUpload"
:onSuccess="onSuccess"
:onError="onError"
:onProgress="onProgress"
>
<a-button>
<a-icon type="upload" /> 选择文件
</a-button>
</a-upload>
</div>
</template>
<script>
import { AUpload } from 'arco-design-vue';
import axios from 'axios';
export default {
components: {
AUpload,
},
data() {
return {
uploadUrl: 'http://your-upload-api-url', // 上传接口地址
};
},
methods: {
beforeUpload(file) {
// 在上传文件之前执行的操作,可以在这里对文件进行校验或者预处理
},
onSuccess(response, file) {
// 上传成功后执行的操作,response 是服务器响应数据,file 是上传的文件对象
},
onError(err, response, file) {
// 上传失败后执行的操作,err 是错误对象,response 是服务器响应数据,file 是上传的文件对象
},
onProgress(event, file, fileList) {
// 上传过程中执行的操作,event 是上传进度事件对象,file 是上传的文件对象,fileList 是上传文件列表
},
},
};
</script>
```
3. 通过设置 a-upload 的属性实现图片上传功能
在模板中,你需要使用 a-upload 组件来实现文件上传功能。a-upload 组件提供了多个属性,包括:
- `action`:上传接口地址,必填项。
- `beforeUpload`:上传文件之前执行的操作,可以在这里对文件进行校验或者预处理。
- `onSuccess`:上传成功后执行的操作,`response` 是服务器响应数据,`file` 是上传的文件对象。
- `onError`:上传失败后执行的操作,`err` 是错误对象,`response` 是服务器响应数据,`file` 是上传的文件对象。
- `onProgress`:上传过程中执行的操作,`event` 是上传进度事件对象,`file` 是上传的文件对象,`fileList` 是上传文件列表。
例如,你可以在 `beforeUpload` 方法中对上传的文件进行校验,代码如下:
```vue
<template>
<div>
<a-upload
:action="uploadUrl"
:beforeUpload="beforeUpload"
:onSuccess="onSuccess"
:onError="onError"
:onProgress="onProgress"
>
<a-button>
<a-icon type="upload" /> 选择文件
</a-button>
</a-upload>
</div>
</template>
<script>
import { AUpload } from 'arco-design-vue';
import axios from 'axios';
export default {
components: {
AUpload,
},
data() {
return {
uploadUrl: 'http://your-upload-api-url', // 上传接口地址
};
},
methods: {
beforeUpload(file) {
const isJPG = file.type === 'image/jpeg';
const isPNG = file.type === 'image/png';
const isLt2M = file.size / 1024 / 1024 < 2;
if (!isJPG && !isPNG) {
this.$message.error('只能上传 JPG 或 PNG 格式的图片');
return false;
}
if (!isLt2M) {
this.$message.error('上传的图片大小不能超过 2MB');
return false;
}
return true;
},
onSuccess(response, file) {
this.$message.success('上传成功');
},
onError(err, response, file) {
this.$message.error('上传失败,请重试');
},
onProgress(event, file, fileList) {
console.log(event, file, fileList);
},
},
};
</script>
```
在 `beforeUpload` 方法中,我们对上传的文件进行了类型和大小的校验,如果不符合要求就提示用户并返回 `false`,否则返回 `true`,继续执行上传操作。
在 `onSuccess` 方法中,我们做了一个简单的提示,告诉用户上传成功了。
在 `onError` 方法中,我们也做了一个简单的提示,告诉用户上传失败了。
在 `onProgress` 方法中,我们将上传进度信息打印到了控制台上,你可以根据实际情况做出对应的操作。
最后,你需要根据实际需求,修改上传接口地址和其他属性,就可以愉快地使用 a-upload 组件实现图片上传功能了。
阅读全文
相关推荐
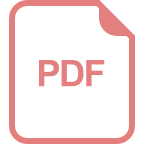

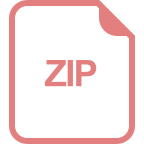
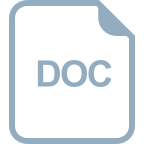
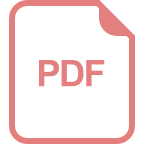
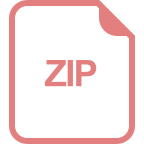
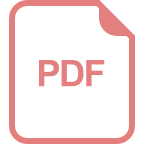
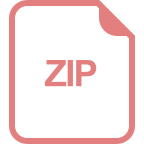
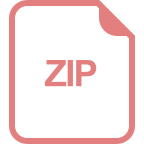
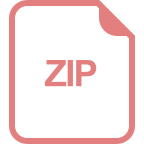
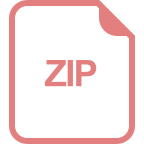
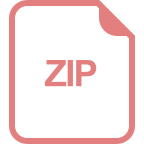
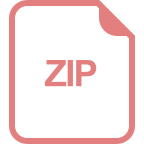
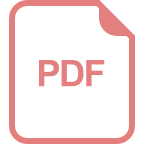
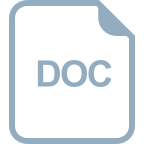
