#include<iostream> #include<iomanip> #include<cstring> using namespace std; struct Student { int num; char name[10]; int score[3]; }; double avgScore(struct Student* p) { double sum = 0; double avg; for (int i = 0; i < 3; i++) { sum += p->score[i]; } avg = sum / 3.0; return avg; } int main() { int n; double avg[50]; cin >> n; struct Student s[50]; for (int i = 0; i < n; i++) { cin >> s[i].num >> s[i].name >> s[i].score[0] >> s[i].score[1] >> s[i].score[2]; } struct Student* p = s; for (int i = 0; i < n; i++) { avg[i] = avgScore(p); p++; } for (int i = 0; i < n; i++) { for (int j = i; j < n; j++) { if (avg[i] < avg[j]){ int temp = avg[i]; struct Student t = s[i]; avg[i] = avg[j]; s[i] = s[j]; avg[j] = temp; s[j] = t; } } } for (int i = 0; i < n; i++) { cout << s[i].num << "," << s[i].name << "," << fixed << setprecision(2) << avg[i] << endl; } }\
时间: 2024-03-30 17:36:41 浏览: 52
这是一段C++代码,用于计算一组学生的平均分,并按平均分从高到低排序,最后输出每个学生的学号、姓名和平均分。具体实现如下:
1. 定义一个结构体Student,包括学号、姓名和三科成绩。
2. 定义一个函数avgScore,用于计算一个学生的平均分。参数是一个指向Student的指针,返回值是这个学生的平均分。
3. 在main函数中,先输入学生的数量n,然后定义一个长度为n的Student数组s,输入每个学生的信息:学号、姓名和三科成绩。
4. 定义一个指向Student的指针p,指向数组s的首地址。
5. 遍历数组s,计算每个学生的平均分,并存储在一个长度为n的double数组avg中。
6. 对学生的平均分从高到低排序,使用冒泡排序算法实现。
7. 遍历排序后的数组s,输出每个学生的学号、姓名和平均分。
这段代码的主要思路是利用结构体存储学生信息,通过指针和数组对学生信息进行操作,最终实现对学生的排序和输出。
相关问题
#include<iostream> #include<cstring> #include<vector> #include<unordered_map> #include<algorithm> #include<queue> #include<iomanip> #include<cmath> #include <fstream> #include <cstdlib> #include <map> // #define int long long using namespace std; const int N = 200010; int n,m,t,p; int ar[N],s[N],cnt,sum,ans; int dx[4]={0,0,1,-1},dy[4]={1,-1,0,0}; double a,b; string st; struct pe { double num,p; }pv[N]; bool cmp (pe a,pe b) { if(a.p==b.p)return a.num<b.num; return a.p>b.p; } void solve () { cin>>n; for(int i=0;i<n;i++) { cin>>a>>b; pv[i].p=a/(a+b); pv[i].num=i+1; } sort(pv,pv+n,cmp); for(int i=0;i<n;i++)cout<<pv[i].num<<" "; } int main() { ios::sync_with_stdio(0); cin.tie(0); cout.tie(0); solve(); return 0; } 请找出代码错误
代码中没有明显的错误。但是,可能存在一些潜在的问题。
1. 在排序函数`cmp`中,当两个`pe`结构体的`p`值相等时,比较的是`num`值。这可能会导致不稳定的排序结果,如果对结果的顺序有要求,可能需要修改排序函数。
2. `pv`数组的大小为`N`,如果`n`的值大于`N`,可能会导致数组越界。
3. 在读取输入时,没有进行输入合法性检查。如果输入的数据格式不正确,可能会导致程序出错。
4. 程序中定义了一些未使用的变量,如`t`、`m`、`s`、`ar`等。可以考虑删除这些无用的变量。
请注意,以上问题只是可能存在的潜在问题,具体还需要根据实际运行情况来确定错误的原因。
#include<iostream> #include<iomanip> #include<cstring> struct Student{ int num; char name[10]; int score[3]; }; int avgScore(struct Student *s){ int sum=0; double avg; for(int i=0;i<3;i++){ sum+=s.score[i]; } avg=(1.0)*sum/3.0; return avg; } int main{ int n; cin>>n; struct Student s[50]; for(int i=0;i<n;i++){ cin>>s[i].num>>s[i].name>>s[i].score[0]>>s[i].score[1]>>s[i].score[2]; } }
首先需要注意的是,在 `avgScore` 函数中,传入的是一个指向结构体 `Student` 的指针,因此在计算成绩平均分时,需要使用箭头符号 `->` 来访问结构体成员的值。
另外,在 `main` 函数中,除了输入学生信息之外,还需要调用 `avgScore` 函数来计算平均分,并输出结果。修改后的代码如下:
```
#include <iostream>
#include <iomanip>
#include <cstring>
using namespace std;
struct Student {
int num;
char name[10];
int score[3];
};
double avgScore(struct Student *s) {
int sum = 0;
double avg;
for (int i = 0; i < 3; i++) {
sum += s->score[i];
}
avg = (1.0) * sum / 3.0;
return avg;
}
int main() {
int n;
cin >> n;
struct Student s[50];
for (int i = 0; i < n; i++) {
cin >> s[i].num >> s[i].name >> s[i].score[0] >> s[i].score[1] >> s[i].score[2];
}
for (int i = 0; i < n; i++) {
cout << s[i].num << " " << s[i].name << " ";
for (int j = 0; j < 3; j++) {
cout << s[i].score[j] << " ";
}
cout << fixed << setprecision(2) << avgScore(&s[i]) << endl;
}
return 0;
}
```
在上述代码中,定义了一个结构体 `Student`,其中包含了学号、姓名和三门课程的成绩。在 `avgScore` 函数中,传入了一个指向结构体 `Student` 的指针,通过箭头符号 `->` 访问结构体成员的值,并计算平均分并返回。在 `main` 函数中,先输入学生信息,然后遍历数组,输出每个学生的学号、姓名、三门课程的成绩和平均分。使用 `fixed` 和 `setprecision` 控制输出小数点后两位。
阅读全文
相关推荐
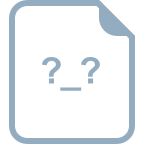




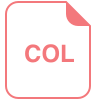


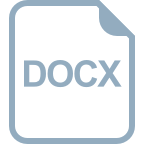
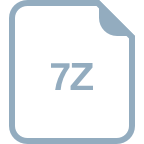
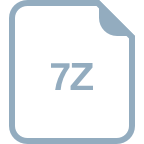
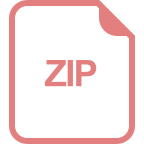