import pandas as pd import requests import xml.etree.ElementTree as ET # 读取 CSV 文件 df = pd.read_csv('coordinates.csv') # 构造请求 URL COORDS = ';'.join([f"{row['latitude']},{row['longitude']}" for index, row in df.iterrows()]) URL = f"http://dev.virtualearth.net/REST/v1/Locations/{COORDS}?o=xml&key=YOUR_BING_MAPS_KEY" # 发送请求 response = requests.get(URL) # 解析响应 root = ET.fromstring(response.content) for location in root.findall(".//{http://schemas.microsoft.com/search/local/ws/rest/v1}Location"): address = location.find("{http://schemas.microsoft.com/search/local/ws/rest/v1}Address") latitude = location.findtext('{http://schemas.microsoft.com/search/local/ws/rest/v1}Point/{http://schemas.microsoft.com/search/local/ws/rest/v1}Latitude') longitude = location.findtext('{http://schemas.microsoft.com/search/local/ws/rest/v1}Point/{http://schemas.microsoft.com/search/local/ws/rest/v1}Longitude') formatted_address = address.findtext('{http://schemas.microsoft.com/search/local/ws/rest/v1}FormattedAddress') print(f"{latitude}, {longitude}: {formatted_address}")修改以上代码,将经纬度以及输出结果都以csv格式保存到桌面
时间: 2023-09-15 11:14:56 浏览: 89
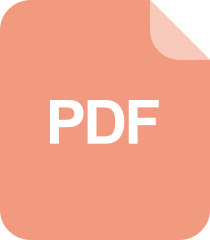
详解pandas库pd.read_excel操作读取excel文件参数整理与实例
import pandas as pd
import requests
import xml.etree.ElementTree as ET
import os
# 读取 CSV 文件
df = pd.read_csv('coordinates.csv')
# 构造请求 URL
COORDS = ';'.join([f"{row['latitude']},{row['longitude']}" for index, row in df.iterrows()])
URL = f"http://dev.virtualearth.net/REST/v1/Locations/{COORDS}?o=xml&key=YOUR_BING_MAPS_KEY"
# 发送请求
response = requests.get(URL)
# 解析响应
root = ET.fromstring(response.content)
results = []
for location in root.findall(".//{http://schemas.microsoft.com/search/local/ws/rest/v1}Location"):
address = location.find("{http://schemas.microsoft.com/search/local/ws/rest/v1}Address")
latitude = location.findtext('{http://schemas.microsoft.com/search/local/ws/rest/v1}Point/{http://schemas.microsoft.com/search/local/ws/rest/v1}Latitude')
longitude = location.findtext('{http://schemas.microsoft.com/search/local/ws/rest/v1}Point/{http://schemas.microsoft.com/search/local/ws/rest/v1}Longitude')
formatted_address = address.findtext('{http://schemas.microsoft.com/search/local/ws/rest/v1}FormattedAddress')
results.append([latitude, longitude, formatted_address])
# 保存结果到CSV文件
df_results = pd.DataFrame(results, columns=['latitude', 'longitude', 'formatted_address'])
df_results.to_csv(os.path.join(os.path.expanduser('~'), 'Desktop', 'results.csv'), index=False)
# 输出结果
print(df_results)
阅读全文
相关推荐
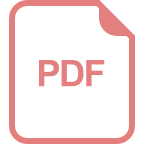

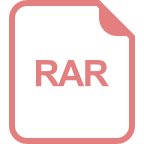
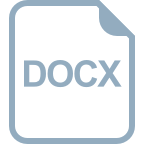
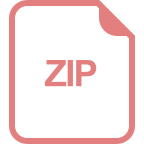
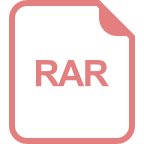
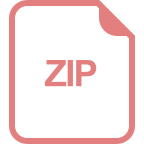
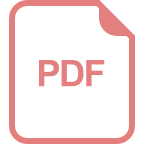
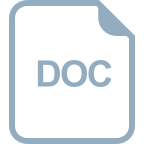
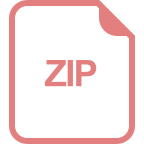