用JavaSocket编程实现白板程序
时间: 2024-03-03 19:50:31 浏览: 138
实现一个基于Java Socket编程的白板程序可以分为以下步骤:
1.创建服务器端和客户端的Socket连接。
2.服务器端需要绑定一个端口号,等待客户端连接。客户端需要连接到服务器端的IP地址和端口号。
3.建立连接后,客户端可以向服务器端发送请求,服务器端可以接收并处理请求。
4.客户端可以通过鼠标事件监听器来获取用户的鼠标操作,然后将操作发送给服务器端。服务器端可以接收并处理这些操作,然后将这些操作广播给所有连接的客户端。
5.客户端接收到广播的操作后,需要将这些操作绘制在画板上。
6.当客户端关闭连接时,需要通知服务器端,服务器端需要将该客户端从连接列表中删除。
7.程序需要考虑多线程并发的情况,以保证程序的稳定性和性能。
具体实现可以参考以下代码:
服务器端:
```
import java.io.*;
import java.net.*;
import java.util.*;
public class Server {
private static final int PORT = 8000;
private static List<Socket> sockets = new ArrayList<Socket>();
public static void main(String[] args) {
try {
ServerSocket ss = new ServerSocket(PORT);
System.out.println("Server started.");
while (true) {
Socket s = ss.accept();
sockets.add(s);
System.out.println("New client connected.");
new Thread(new ClientHandler(s)).start();
}
} catch (IOException e) {
e.printStackTrace();
}
}
static class ClientHandler implements Runnable {
Socket s;
String name;
public ClientHandler(Socket s) {
this.s = s;
}
public void run() {
try {
BufferedReader in = new BufferedReader(new InputStreamReader(s.getInputStream()));
PrintWriter out = new PrintWriter(new OutputStreamWriter(s.getOutputStream()), true);
out.println("Welcome to the whiteboard!");
while (true) {
String line = in.readLine();
if (line == null) {
break;
}
if (name == null) { // the first line is the name
name = line;
} else {
broadcast(name + ": " + line);
}
}
System.out.println("Client disconnected.");
sockets.remove(s);
s.close();
} catch (IOException e) {
e.printStackTrace();
}
}
private void broadcast(String message) {
for (Socket s : sockets) {
try {
PrintWriter out = new PrintWriter(new OutputStreamWriter(s.getOutputStream()), true);
out.println(message);
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
```
客户端:
```
import java.awt.*;
import java.awt.event.*;
import java.io.*;
import java.net.*;
import javax.swing.*;
public class Client extends JFrame {
private static final long serialVersionUID = 1L;
private static final String SERVER_ADDRESS = "localhost";
private static final int PORT = 8000;
private static final int WIDTH = 800;
private static final int HEIGHT = 600;
private Socket s;
private BufferedReader in;
private PrintWriter out;
private Whiteboard whiteboard;
public Client() {
super("Whiteboard");
try {
s = new Socket(SERVER_ADDRESS, PORT);
in = new BufferedReader(new InputStreamReader(s.getInputStream()));
out = new PrintWriter(new OutputStreamWriter(s.getOutputStream()), true);
String welcomeMessage = in.readLine();
System.out.println(welcomeMessage);
String name = JOptionPane.showInputDialog("Enter your name:");
out.println(name);
whiteboard = new Whiteboard();
add(whiteboard);
new Thread(new Receiver()).start();
setSize(WIDTH, HEIGHT);
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
} catch (IOException e) {
e.printStackTrace();
}
}
private class Receiver implements Runnable {
public void run() {
try {
while (true) {
String message = in.readLine();
whiteboard.append(message);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
s.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
private class Whiteboard extends JPanel implements MouseListener, MouseMotionListener {
private static final long serialVersionUID = 1L;
private StringBuilder sb = new StringBuilder();
private int lastX, lastY;
public Whiteboard() {
setBackground(Color.WHITE);
addMouseListener(this);
addMouseMotionListener(this);
}
public void paintComponent(Graphics g) {
super.paintComponent(g);
g.setColor(Color.BLACK);
g.drawString(sb.toString(), 20, 20);
}
public void append(String message) {
sb.append(message + "\n");
repaint();
}
public void mousePressed(MouseEvent e) {
lastX = e.getX();
lastY = e.getY();
}
public void mouseDragged(MouseEvent e) {
int x = e.getX();
int y = e.getY();
out.println("LINE " + lastX + " " + lastY + " " + x + " " + y);
lastX = x;
lastY = y;
}
public void mouseClicked(MouseEvent e) {}
public void mouseEntered(MouseEvent e) {}
public void mouseExited(MouseEvent e) {}
public void mouseReleased(MouseEvent e) {}
public void mouseMoved(MouseEvent e) {}
}
public static void main(String[] args) {
new Client();
}
}
```
阅读全文
相关推荐
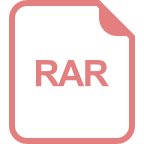




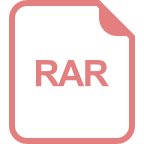
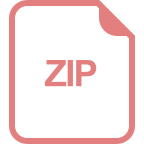
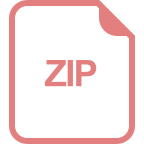


