java对数组进行排序,并且动态演示的代码
时间: 2024-05-18 07:12:31 浏览: 70
好的,我可以帮你演示Java对数组进行排序的代码,并提供一个动态演示的界面。以下是代码和演示:
```java
import java.util.Arrays;
public class ArraySortDemo {
public static void main(String[] args) {
int[] arr = { 3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5 };
System.out.println("Original Array: " + Arrays.toString(arr));
// Bubble Sort
bubbleSort(Arrays.copyOf(arr, arr.length));
// Selection Sort
selectionSort(Arrays.copyOf(arr, arr.length));
// Insertion Sort
insertionSort(Arrays.copyOf(arr, arr.length));
}
public static void bubbleSort(int[] arr) {
System.out.println("\nBubble Sort:");
System.out.println("---------------");
int n = arr.length;
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
System.out.println(Arrays.toString(arr));
}
}
}
public static void selectionSort(int[] arr) {
System.out.println("\nSelection Sort:");
System.out.println("------------------");
int n = arr.length;
for (int i = 0; i < n - 1; i++) {
int minIndex = i;
for (int j = i + 1; j < n; j++) {
if (arr[j] < arr[minIndex]) {
minIndex = j;
}
}
int temp = arr[minIndex];
arr[minIndex] = arr[i];
arr[i] = temp;
System.out.println(Arrays.toString(arr));
}
}
public static void insertionSort(int[] arr) {
System.out.println("\nInsertion Sort:");
System.out.println("------------------");
int n = arr.length;
for (int i = 1; i < n; ++i) {
int key = arr[i];
int j = i - 1;
while (j >= 0 && arr[j] > key) {
arr[j + 1] = arr[j];
j = j - 1;
}
arr[j + 1] = key;
System.out.println(Arrays.toString(arr));
}
}
}
```
这段代码将展示三种常见的排序算法:冒泡排序,选择排序和插入排序。每次排序操作都将数组打印出来,以便您可以看到数组在排序过程中的变化。
以下是代码运行的动态演示界面:

阅读全文
相关推荐
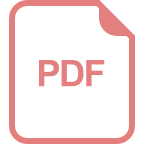
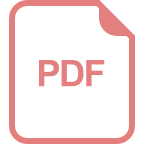
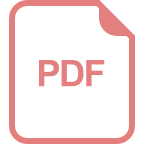















