c++程序:用vector容器储存城市的历史人口信息,并可以进行添加,删除,排序,查找等功能
时间: 2024-05-12 13:13:23 浏览: 41
以下是一个使用vector容器储存城市历史人口信息的示例程序,程序中实现了添加、删除、排序和查找等功能:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
struct City {
string name;
int population;
bool operator<(const City &other) const {
return name < other.name;
}
};
void printCity(const City &city) {
cout << "City name: " << city.name << ", population: " << city.population << endl;
}
void addCity(vector<City> &cities) {
cout << "Enter city name: ";
string name;
getline(cin, name);
cout << "Enter population: ";
int population;
cin >> population;
cin.ignore(); // Ignore the newline character left in the input buffer after reading population
City city = {name, population};
cities.push_back(city);
cout << "City added successfully!" << endl;
}
void deleteCity(vector<City> &cities) {
cout << "Enter city name to delete: ";
string name;
getline(cin, name);
auto it = find_if(cities.begin(), cities.end(), [&](const City &city) {
return city.name == name;
});
if (it != cities.end()) {
cities.erase(it);
cout << "City deleted successfully!" << endl;
} else {
cout << "City not found!" << endl;
}
}
void sortCities(vector<City> &cities) {
sort(cities.begin(), cities.end());
cout << "Cities sorted by name!" << endl;
}
void searchCity(vector<City> &cities) {
cout << "Enter city name to search: ";
string name;
getline(cin, name);
auto it = find_if(cities.begin(), cities.end(), [&](const City &city) {
return city.name == name;
});
if (it != cities.end()) {
printCity(*it);
} else {
cout << "City not found!" << endl;
}
}
int main() {
vector<City> cities;
while (true) {
cout << "Select an option:" << endl;
cout << "1. Add city" << endl;
cout << "2. Delete city" << endl;
cout << "3. Sort cities by name" << endl;
cout << "4. Search city" << endl;
cout << "5. Exit" << endl;
int option;
cin >> option;
cin.ignore(); // Ignore the newline character left in the input buffer after reading option
switch (option) {
case 1:
addCity(cities);
break;
case 2:
deleteCity(cities);
break;
case 3:
sortCities(cities);
break;
case 4:
searchCity(cities);
break;
case 5:
cout << "Goodbye!" << endl;
return 0;
default:
cout << "Invalid option! Please try again." << endl;
break;
}
cout << endl;
}
return 0;
}
```
在程序中,我们定义了一个名为`City`的结构体,其中包含了城市的名称和人口。为了实现按照城市名称排序的功能,我们在结构体中重载了小于号运算符。
在`addCity`函数中,我们使用了`getline`函数来读取用户输入的字符串,并使用`cin.ignore`函数来忽略读取人口数后留在输入缓冲区中的换行符。在`deleteCity`和`searchCity`函数中,我们使用了`find_if`算法来查找城市。
在程序的主函数中,我们使用了一个无限循环来接受用户的操作选择,并根据用户的选择调用相应的函数。
阅读全文
相关推荐
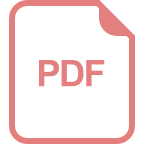
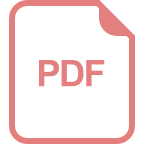
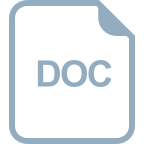
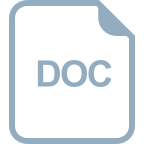
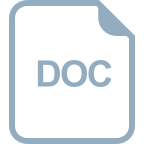
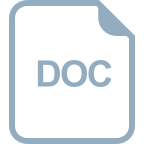



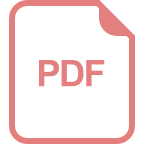
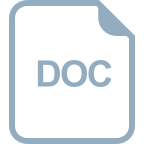
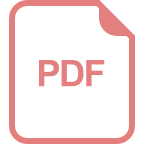
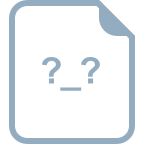
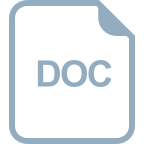
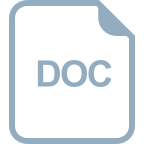
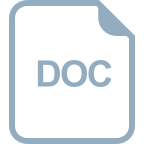
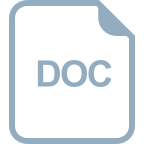