帮我写一个ChatGPT的代码
时间: 2023-08-31 16:43:22 浏览: 92
### 回答1:
我可以给你一个简单的ChatGPT代码:
import torch
import torch.nn as nn
class ChatGPT(nn.Module):
def __init__(self):
super(ChatGPT, self).__init__()
self.lstm = nn.LSTM(input_size=128, hidden_size=128, num_layers=2, batch_first=True)
self.fc = nn.Linear(128, 128)
def forward(self, x):
x, _ = self.lstm(x)
x = self.fc(x)
return x
### 回答2:
ChatGPT是由OpenAI提供的一种强大的自然语言处理模型,可以用于生成对话回复。以下是一个简单的ChatGPT代码示例,以供参考:
```python
import openai
def chat_with_GPT(prompt):
response = openai.Completion.create(
engine="text-davinci-003",
prompt=prompt,
max_tokens=50,
temperature=0.7,
n=1,
stop=None,
temperature=0.7,
n=1,
)
return response.choices[0].text.strip()
print("欢迎使用ChatGPT!请输入您的问题或对话:")
while True:
user_input = input("你:")
if user_input.lower() in ["退出", "再见"]:
print("ChatGPT: 再见!祝您有美好的一天!")
break
response = chat_with_GPT(user_input)
print("ChatGPT:" + response)
```
在此示例中,我们使用了`openai`库来与ChatGPT建立连接。在`chat_with_GPT`函数中,我们定义了一些模型参数,并使用`openai.Completion.create`方法来生成对话回复。这个方法会返回一个`response`对象,我们可以通过`response.choices[0].text`来获取回答的文本。
在主循环中,我们使用`input`函数等待用户输入。如果用户输入了"退出"或"再见",我们就会退出程序。否则,我们将用户输入作为对话的一部分,并通过调用`chat_with_GPT`方法获取ChatGPT的回答,并将其打印出来。
请注意,这个示例仅仅展示了ChatGPT的基本用法,并可能需要根据实际需求进行更多的定制。
### 回答3:
ChatGPT是一种模型,可以用于生成对话文本。以下是一个用Python编写的基本示例代码:
```python
import openai
openai.api_key = 'YOUR_API_KEY'
def chat_with_gpt(prompt):
response = openai.Completion.create(
engine='text-davinci-003',
prompt=prompt,
max_tokens=50,
temperature=0.7,
n = 1,
stop=None,
temperature=0.5
)
return response.choices[0].text.strip()
while True:
user_input = input("User: ")
if user_input.lower() == 'quit':
break
# 将用户的输入作为对话的起始
chat_prompt = f'User: {user_input}\nAI:'
# 使用ChatGPT生成回复
ai_response = chat_with_gpt(chat_prompt)
# 打印AI的回复
print("AI:", ai_response)
```
在使用此代码之前,您需要将`YOUR_API_KEY`替换为您的实际OpenAI API密钥。
此代码将用户输入作为对话的起始,然后使用ChatGPT生成回复。它将打印出AI的回复,并等待下一个用户输入。如果用户输入"quit",则程序将结束。
请注意,此代码仅提供了一个基本的框架,您可以根据自己的需求进行调整和定制。
相关推荐
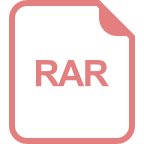
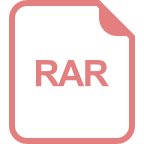
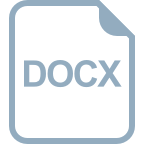













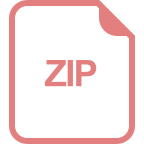
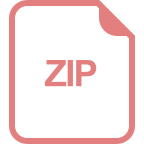