python如何resize二维数组
时间: 2023-05-31 19:05:11 浏览: 176
可以使用numpy库中的resize函数实现二维数组的resize操作,示例如下:
```python
import numpy as np
arr = np.array([[1, 2], [3, 4]])
# 将二维数组resize为3行3列
resized_arr = np.resize(arr, (3, 3))
print(resized_arr)
```
输出结果为:
```
array([[1, 2, 3],
[4, 1, 2],
[3, 4, 1]])
```
其中,resize函数的第一个参数为原始二维数组,第二个参数为resize后的形状,这里将原始数组resize为3行3列,多余的元素会从头开始重复填充。
相关问题
np0维数组变成一维数组
要将0维数组变成一维数组,可以使用numpy的reshape函数或resize函数。引用\[2\]中的代码演示了如何使用reshape函数将一维数组变成4行5列的二维数组。你可以根据需要修改代码中的参数来适应你的情况。另外,你也可以使用resize函数来覆盖原来的数组,将新的结果给原来的数组,从而实现将0维数组变成一维数组。请参考引用\[2\]中的代码示例。
#### 引用[.reference_title]
- *1* *3* [python numpy 一维数组转变为多维数组的实例](https://blog.csdn.net/weixin_33657499/article/details/114930344)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down1,239^v3^insert_chatgpt"}} ] [.reference_item]
- *2* [Python数组操作将一维数组变成二维数组](https://blog.csdn.net/qq_40185847/article/details/114446896)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^insert_down1,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
有若干图像,进行读取,转为二维数组,将图片像素值归一化到0~1,改变图片尺寸为 [N, C, H, W],,获取图像一维标签
,最终返回由图像二维数组和对应标签组成的数据集。
以下是Python代码示例:
```python
import os
import numpy as np
from PIL import Image
def read_images(data_path, img_size):
images = []
labels = []
for foldername in os.listdir(data_path):
folderpath = os.path.join(data_path, foldername)
if os.path.isdir(folderpath):
for filename in os.listdir(folderpath):
if filename.endswith(".jpg"):
filepath = os.path.join(folderpath, filename)
img = Image.open(filepath).convert("L") # 转为灰度图
img = img.resize(img_size) # 改变图片尺寸
img_arr = np.array(img) / 255.0 # 归一化到0~1
images.append(img_arr)
labels.append(int(foldername))
images = np.array(images)
labels = np.array(labels)
return images, labels
data_path = "path/to/images"
img_size = (28, 28) # 图像尺寸
images, labels = read_images(data_path, img_size)
# 转为 [N, C, H, W] 形式
images = images.reshape(images.shape[0], 1, img_size[0], img_size[1])
# 返回数据集
dataset = {"images": images, "labels": labels}
```
阅读全文
相关推荐
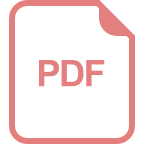
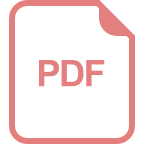
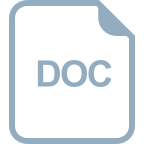
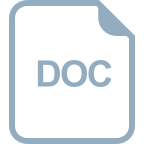
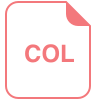
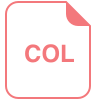

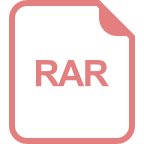
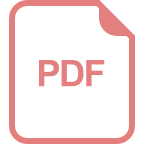
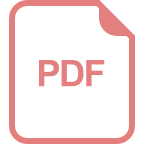
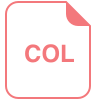



