python 怎么定义异步服务grpc
时间: 2024-12-19 08:22:10 浏览: 30
在Python中,使用gRPC(Google Remote Procedure Call)定义异步服务通常需要以下几个步骤:
1. **安装依赖**:
首先确保已经安装了gRPC和protobuf库,可以用pip安装:
```
pip install grpcio protobuf
```
2. **定义.proto文件**:
创建一个`.proto`协议缓冲文件来描述服务接口和服务消息结构。例如:
```proto
syntax = "proto3";
service AsyncService {
rpc SayHello (HelloRequest) returns (HelloReply);
}
message HelloRequest {
string name = 1;
}
message HelloReply {
string message = 1;
}
```
3. **生成Python代码**:
使用protoc工具(protoc-gen-grpc-python插件)从`.proto`文件生成Python代码:
```
protoc -I=$SRC_DIR --python_out=$DST_DIR --grpc_python_out=$DST_DIR $SRC_DIR/your_service.proto
```
其中$SRC_DIR和$DST_DIR分别是包含.proto文件和存放生成代码的位置。
4. **定义服务端**:
在Python中,创建一个实现了`AsyncService`接口的服务类,并添加异步方法:
```python
import concurrent.futures
from concurrent.futures import ThreadPoolExecutor
import your_service_pb2_grpc as grpc_service # 自动导入由protoc生成的库
class AsyncServiceImpl(grpc_service.AsyncServiceServicer):
def __init__(self):
self.executor = ThreadPoolExecutor()
async def SayHello(self, request, context):
# 异步处理请求,这里仅作示例,实际应用可能需要数据库查询或其他耗时操作
response = your_service_pb2.HelloReply(message="Hello, " + request.name)
await self.executor.submit(self._do_something, request, response)
return response
async def _do_something(self, request, reply):
# 执行耗时操作,然后更新reply
time.sleep(1)
reply.message += ", world!"
server = grpc.server(futures.ThreadPoolExecutor(max_workers=10))
grpc_service.add_AsyncServiceServicer_to_server(AsyncServiceImpl(), server)
server.add_insecure_port('[::]:50051') # 配置服务监听地址
server.start() # 启动服务器
server.wait_for_termination() # 等待服务器关闭
```
5. **客户端连接**:
使用gRPC客户端库调用异步服务:
```python
from your_service_pb2 import HelloRequest
from your_service_pb2_grpc import AsyncServiceStub
async def call_async_service():
stub = AsyncServiceStub(channel)
request = HelloRequest(name="Client")
response = await stub.SayHello(request)
print(response.message)
asyncio.run(call_async_service()) # 异步调用
```
阅读全文
相关推荐


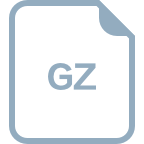










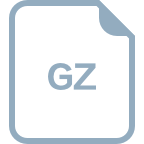

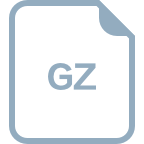


