编写一个Java程序,实现以下指定功能: 1) 将HDFS中指定文件的内容输出到终端中,如果指定文件不存在要给出提示; 2) 向HDFS中指定的文件追加内容,由用户指定内容追加到原有文件的开头或结尾,追加后的文件可以保存为其他名字; 将代码和运行情况截图上传
时间: 2024-10-19 13:06:41 浏览: 66
在Java中操作Hadoop HDFS通常需要使用Hadoop的API,如Hadoop File System (HDFS) API。这里我会提供一个基本的示例代码框架,但实际上你需要引入`hadoop-client`库,并配置好Hadoop环境。
首先,确保已添加了Hadoop的相关依赖,然后你可以编写如下代码:
```java
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
import java.io.IOException;
import java.util.Scanner;
public class HdfsOperations {
public static void main(String[] args) {
// 初始化HDFS配置
Configuration conf = new Configuration();
try {
FileSystem fs = FileSystem.get(conf);
// 检查并读取文件是否存在
String filePath = "hdfs://your_hdfs_path/your_file.txt"; // 替换为实际HDFS路径
Path path = new Path(filePath);
if (!fs.exists(path)) {
System.out.println("File does not exist in HDFS.");
return;
}
// 输出文件内容到终端
System.out.println("Reading file content:");
byte[] buffer = new byte[1024];
int bytesRead;
InputStream inputStream = fs.open(path);
while ((bytesRead = inputStream.read(buffer)) != -1) {
System.out.write(buffer, 0, bytesRead);
}
inputStream.close();
// 追加内容到文件
Scanner scanner = new Scanner(System.in);
System.out.println("Enter the content to append (start or end):");
String mode = scanner.nextLine().toLowerCase();
if ("start".equals(mode)) {
appendContentToStart(fs, path);
} else if ("end".equals(mode)) {
appendContentToEnd(fs, path);
} else {
System.out.println("Invalid option, appending to end by default.");
appendContentToEnd(fs, path);
}
// 另存文件(如果需要)
String newFileName = "new_file.txt";
fs.rename(path, new Path("/path/to/" + newFileName)); // 替换为新的HDFS路径
fs.close();
} catch (IOException e) {
e.printStackTrace();
}
}
private static void appendContentToStart(FileSystem fs, Path path) throws IOException {
DataOutputStream outputStream = fs.append(path);
outputStream.writeBytes("Appended at start.\n");
outputStream.close();
}
private static void appendContentToEnd(FileSystem fs, Path path) throws IOException {
DataOutputStream outputStream = fs.create(path, true); // 'true' means append
outputStream.writeBytes("Appended at end.\n");
outputStream.close();
}
}
```
请确保将`your_hdfs_path`、`your_file.txt`以及最后的`new_file.txt`替换为你实际的HDFS路径和文件名。运行这个程序时,它会先检查文件是否存在,然后读取并打印其内容,接着让用户输入追加内容的位置(开始或结束),然后执行相应的追加操作,并保存为新文件。
注意:在实际生产环境中,你应该处理可能出现的所有异常,并且可能需要更复杂的权限管理。此外,Hadoop的客户端操作最好在一个受Hadoop守护进程守护的安全上下文中进行,而不是直接在Java应用程序中。
阅读全文
相关推荐
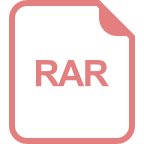
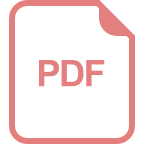
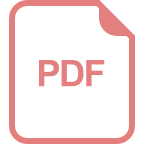




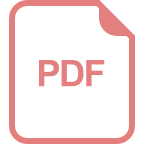
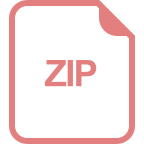
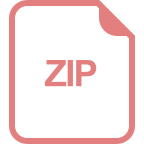
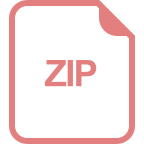
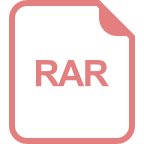
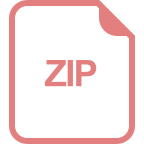
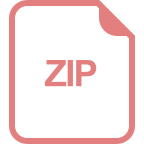
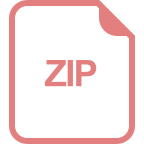
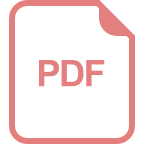
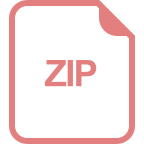
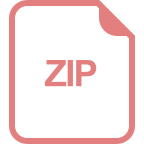