python_gnupg中对文件进行压缩并对压缩后的文件进行对称加密算法进行加密
时间: 2024-03-27 13:39:34 浏览: 14
可以使用`python_gnupg`库来实现对文件进行压缩并对压缩后的文件进行对称加密算法进行加密。下面是一个简单的示例代码:
```python
import gnupg
import gzip
import os
# 创建GPG对象
gpg = gnupg.GPG()
# 设置对称加密算法
gpg.encoding = 'utf-8'
gpg.options.symmetric = 'AES256'
# 原始文件路径和名称
file_path = '/path/to/your/file'
file_name = 'your_file.txt'
# 压缩文件路径和名称
compressed_file_path = '/path/to/your/compressed/file'
compressed_file_name = 'your_file.txt.gz'
# 加密文件路径和名称
encrypted_file_path = '/path/to/your/encrypted/file'
encrypted_file_name = 'your_file.txt.gz.gpg'
# 压缩文件
with open(file_path + '/' + file_name, 'rb') as f_in:
with gzip.open(compressed_file_path + '/' + compressed_file_name, 'wb') as f_out:
f_out.writelines(f_in)
# 加密文件
with open(compressed_file_path + '/' + compressed_file_name, 'rb') as f:
status = gpg.encrypt_file(
f,
recipients=None, # 如果不指定接收者,则使用对称加密
output=encrypted_file_path + '/' + encrypted_file_name
)
# 删除临时文件
os.remove(compressed_file_path + '/' + compressed_file_name)
if status.ok:
print('File encrypted successfully!')
else:
print('Encryption failed!')
```
在上面的示例代码中,我们首先创建了一个`GPG`对象,并设置了对称加密算法为`AES256`。然后我们指定了原始文件的路径和名称,压缩文件的路径和名称,以及加密文件的路径和名称。接着我们使用`gzip`模块对原始文件进行压缩,将压缩后的文件传递给`gpg.encrypt_file()`方法进行加密。加密成功后,我们删除了临时的压缩文件。最后,我们检查了加密的状态并输出结果。
相关推荐
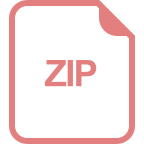
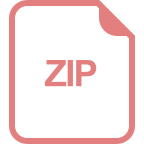
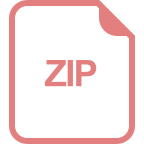














