<mapper namespace="com.cskaoyan.mapper.MarketOrderMapper"> <resultMap id="BaseResultMap" type="com.cskaoyan.bean.MarketOrder"> <id column="id" jdbcType="INTEGER" property="id" /> <result column="user_id" jdbcType="INTEGER" property="userId" /> <result column="order_sn" jdbcType="VARCHAR" property="orderSn" /> <result column="order_status" jdbcType="SMALLINT" property="orderStatus" /> <result column="aftersale_status" jdbcType="SMALLINT" property="aftersaleStatus" /> <result column="consignee" jdbcType="VARCHAR" property="consignee" /> <result column="mobile" jdbcType="VARCHAR" property="mobile" /> <result column="address" jdbcType="VARCHAR" property="address" /> <result column="message" jdbcType="VARCHAR" property="message" /> <result column="goods_price" jdbcType="DECIMAL" property="goodsPrice" /> <result column="freight_price" jdbcType="DECIMAL" property="freightPrice" /> <result column="coupon_price" jdbcType="DECIMAL" property="couponPrice" /> <result column="integral_price" jdbcType="DECIMAL" property="integralPrice" /> <result column="groupon_price" jdbcType="DECIMAL" property="grouponPrice" /> <result column="order_price" jdbcType="DECIMAL" property="orderPrice" /> <result column="actual_price" jdbcType="DECIMAL" property="actualPrice" /> <result column="pay_id" jdbcType="VARCHAR" property="payId" /> <result column="pay_time" jdbcType="TIMESTAMP" property="payTime" /> <result column="ship_sn" jdbcType="VARCHAR" property="shipSn" /> <result column="ship_channel" jdbcType="VARCHAR" property="shipChannel" /> <result column="ship_time" jdbcType="TIMESTAMP" property="shipTime" /> <result column="refund_amount" jdbcType="DECIMAL" property="refundAmount" /> <result column="refund_type" jdbcType="VARCHAR" property="refundType" /> <result column="refund_content" jdbcType="VARCHAR" property="refundContent" /> <result column="refund_time" jdbcType="TIMESTAMP" property="refundTime" /> <result column="confirm_time" jdbcType="TIMESTAMP" property="confirmTime" /> <result column="comments" jdbcType="SMALLINT" property="comments" /> <result column="end_time" jdbcType="TIMESTAMP" property="endTime" /> <result column="add_time" jdbcType="TIMESTAMP" property="addTime" /> <result column="update_time" jdbcType="TIMESTAMP" property="updateTime" /> <result column="deleted" jdbcType="BIT" property="deleted" /> </resultMap>
时间: 2023-07-02 12:19:18 浏览: 82
这段代码是一个MyBatis的XML文件中的mapper标签,用于配置与MarketOrder相关的SQL语句和映射关系。其中,resultMap标签用于定义查询结果与Java对象之间的映射关系。具体来说,定义了MarketOrder类的各个属性与查询结果中对应字段的映射关系。例如,id属性对应查询结果中的id字段,userId属性对应查询结果中的user_id字段,以此类推。
在实际的SQL查询中,可以通过引用该resultMap来获取查询结果并将其转换为对应的Java对象,例如:
```
<select id="getOrderById" resultMap="BaseResultMap">
SELECT * FROM market_order WHERE id = #{id}
</select>
```
这里的getOrderById是一个SQL查询语句的ID,resultMap="BaseResultMap"表示将查询结果使用上面定义的BaseResultMap映射到MarketOrder对象中。
相关问题
<mapper namespace="com.example.mapper.AuthorMapper"> </mapper>
这是一个 MyBatis 的 Mapper 文件中的命名空间。命名空间是为了标识这个 Mapper 接口所对应的 SQL 映射文件,方便 MyBatis 进行管理和映射。在命名空间中,可以包含多个 SQL 语句的映射,这些 SQL 语句可以是增删改查等操作。通过命名空间和对应的 Mapper 接口方法名,可以方便地调用 Mapper 中定义的 SQL 语句。
<mapper namespace="com.example.mapper.TrendMapper">
这是一个 MyBatis 的配置文件中的一行代码,它指定了一个命名空间为 "com.example.mapper.TrendMapper" 的 Mapper 接口。在这个命名空间下,可以定义一些 SQL 语句,以及与之对应的方法。这样,在 Java 代码中,就可以通过 MyBatis 的 SqlSession 对象调用这些方法,从而执行 SQL 查询、插入、更新等操作。与前一个问题中的代码类似,只是命名空间不同。
相关推荐
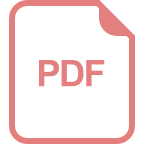
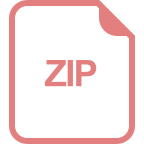
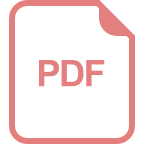













