c++ pta 带头单链表实现 求表长,定位和插入三个函数
时间: 2024-10-04 08:01:02 浏览: 44
在 C++ 中,带头结点的单链表是一种常见的数据结构,它的头部有一个额外的节点,用于方便地表示链表的开始。下面是使用 C++ 实现求链表长度、定位元素以及插入元素这三个基本操作的简单示例:
```cpp
// 定义链表节点
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(nullptr) {} // 构造函数
};
// 函数:获取链表长度
size_t getLength(ListNode* head) {
size_t length = 0; // 初始化长度为0
if (head != nullptr) { // 非空链表
ListNode* current = head;
while (current->next != nullptr) { // 遍历整个链表
length++;
current = current->next;
}
}
return length;
}
// 函数:定位指定值的节点
ListNode* findPosition(ListNode* head, int target) {
ListNode* current = head;
while (current != nullptr && current->val != target) {
current = current->next;
}
return current;
}
// 函数:在链表中插入元素(在给定位置)
void insertAtPosition(ListNode** head, int position, int value) {
if (position <= 0 || *head == nullptr) {
// 如果位置错误或链表为空,则在开头插入
ListNode newNode(value);
newNode.next = *head;
*head = &newNode;
return;
}
ListNode* prev = *head;
ListNode* curr = prev->next;
for (int i = 1; i < position - 1 && curr != nullptr; ++i) {
prev = curr;
curr = curr->next;
}
if (curr == nullptr) {
// 如果已到达链表末尾,在末尾插入
prev->next = new ListNode(value);
} else {
// 插入到中间位置
newNode = new ListNode(value);
newNode->next = curr->next;
prev->next = newNode;
}
}
```
阅读全文
相关推荐



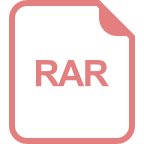




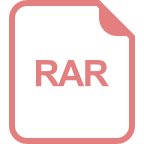
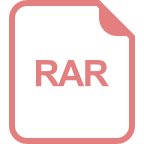

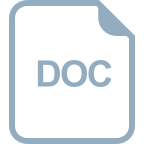