使用Python,找出CSV文件中全部列中的大于70小于80的数字并将其替换为-1,并另存
时间: 2024-12-25 16:29:27 浏览: 3
要实现这个功能,你可以使用Python的`csv`模块来读取CSV文件,`pandas`库来处理数据,以及`openpyxl`(如果文件是Excel格式)或`xlrd`和`xlwt`(如果文件是旧版本的xls格式)来处理Excel文件。这里假设我们正在处理CSV文件:
```python
import csv
import pandas as pd
# 读取CSV文件
def read_csv_replace_values(filename):
# 创建一个临时DataFrame来存储数据
temp_df = pd.read_csv(filename)
# 使用条件筛选和替换
temp_df = temp_df.replace((temp_df > 70) & (temp_df < 80), -1, inplace=True)
# 写回CSV文件
temp_df.to_csv('output.csv', index=False)
# 调用函数处理特定CSV文件
read_csv_replace_values('input.csv')
```
如果你要处理的是Excel文件,可以使用`openpyxl`或`xlrd`/`xlwt`:
```python
from openpyxl import load_workbook
# 处理Excel文件(仅适用于.xlsx格式)
def process_excel(filename):
wb = load_workbook(filename)
ws = wb.active
# 获取最大行数和列数
last_row = ws.max_row
last_column = ws.max_column
for row in range(1, last_row + 1): # 忽略表头
for col in range(1, last_column + 1): # 从第二列开始,因为第一列通常用于标题
cell_value = ws.cell(row=row, column=col).value
if isinstance(cell_value, (int, float)) and 70 < cell_value < 80:
ws.cell(row=row, column=col).value = -1
# 保存更改
wb.save('output.xlsx')
# 对于.xls文件,可以使用xlrd和xlwt
def process_excel_xls(filename):
book = xlrd.open_workbook(filename)
sheet = book.sheet_by_index(0) # 假设默认工作表是第一个
for row in range(sheet.nrows):
for col in range(1, sheet.ncols): # 从第二列开始
cell_value = sheet.cell_value(row, col)
if isinstance(cell_value, (int, float)) and 70 < cell_value < 80:
sheet.write(row, col, -1)
# 写入新文件
new_book = xlwt.Workbook()
new_sheet = new_book.add_sheet('Sheet 1')
new_book.save('output.xls')
# 根据文件扩展名选择相应的方法
filename = 'example_file.xlsx' # 替换为你的文件名
if filename.endswith('.xlsx'):
process_excel(filename)
elif filename.endswith('.xls'):
process_excel_xls(filename)
else:
raise ValueError("Unsupported file format")
```
阅读全文
相关推荐
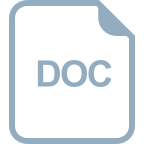
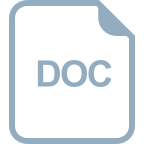
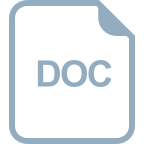
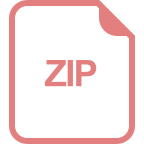
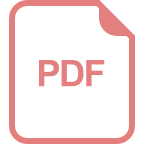
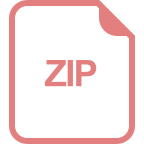
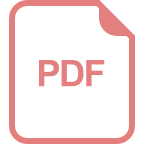
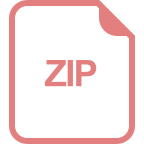
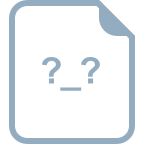
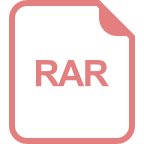
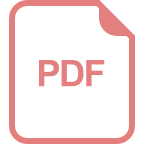
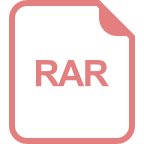
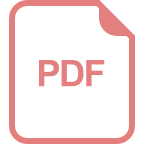
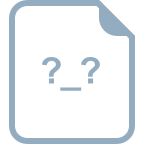
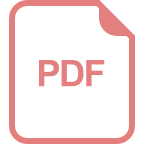