qt获取linux系统窗口
时间: 2023-09-03 21:07:24 浏览: 114
在 Qt 中,可以使用 QX11Info 类获取 Linux 系统窗口的信息。具体步骤如下:
1. 引入 QX11Info 类:
```
#include <QX11Info>
```
2. 获取窗口 ID:
```
WId windowId = QApplication::desktop()->winId();
```
3. 使用 QX11Info 类获取窗口信息:
```
QX11Info xInfo = QX11Info::appRootWindow(QX11Info::appScreen());
Window window = xInfo.xcbConnection()->nativeWindow();
```
其中,`window` 就是 Linux 系统窗口的 ID。
需要注意的是,上述代码只能在 Linux 平台上使用,如果在 Windows 或 macOS 上运行,可能会出现错误。
相关问题
qt获取linux系统所有的窗口
要获取Linux系统中所有窗口的列表,你可以使用X Window系统提供的Xlib库。下面是获取窗口列表的基本步骤:
1. 连接X服务器
```
Display* display = XOpenDisplay(NULL);
```
2. 获取根窗口
```
Window root = DefaultRootWindow(display);
```
3. 获取子窗口列表
```
Window parent;
Window* children;
unsigned int nchildren;
XQueryTree(display, root, &root, &parent, &children, &nchildren);
```
4. 遍历子窗口列表
```
for (unsigned int i = 0; i < nchildren; i++) {
// 处理子窗口
// ...
}
// 释放子窗口列表
XFree(children);
```
在遍历子窗口列表时,你可以使用XGetWindowAttributes函数获取每个窗口的属性,包括窗口的位置、大小和标题等信息。例如,要获取窗口的标题,可以使用以下代码:
```
XTextProperty prop;
if (XGetWMName(display, child, &prop) != 0 && prop.value != NULL) {
char** list;
int count;
XmbTextPropertyToTextList(display, &prop, &list, &count);
if (count > 0) {
// 窗口标题
char* title = list[0];
}
XFreeStringList(list);
XFree(prop.value);
}
```
需要注意的是,由于X Window系统的复杂性,获取窗口信息可能会涉及到许多细节和特殊情况。因此,建议你在编写代码时仔细阅读Xlib库的文档,以确保代码的正确性和可靠性。
qt获取linux系统所有的窗口的示例代码
以下是获取Linux系统所有窗口的示例代码,使用Qt编写:
```cpp
#include <QCoreApplication>
#include <QDebug>
#include <QX11Info>
#include <X11/Xlib.h>
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
Display *display = QX11Info::display();
// 获取默认屏幕
int screen = DefaultScreen(display);
// 获取根窗口
Window root = RootWindow(display, screen);
// 获取所有子窗口
Window parent;
Window *children = NULL;
unsigned int nchildren;
XQueryTree(display, root, &root, &parent, &children, &nchildren);
for (unsigned int i = 0; i < nchildren; i++) {
char *name = NULL;
XFetchName(display, children[i], &name);
if (name != NULL && strlen(name) > 0) {
qDebug() << "Window title:" << name;
XFree(name);
}
}
if (children != NULL) {
XFree(children);
}
return a.exec();
}
```
该代码使用X11库中的函数来获取Linux系统中所有窗口的信息。其中,XQueryTree函数用于获取子窗口,XFetchName函数用于获取窗口标题。
相关推荐
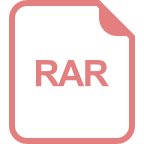












