上传图片预览功能图片功能实现Java
时间: 2025-01-05 09:40:23 浏览: 8
在Java中实现上传图片预览功能,通常需要结合前端和后端技术。以下是一个简单的实现方案:
### 前端实现
前端可以使用HTML和JavaScript来实现图片上传和预览功能。
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>图片上传预览</title>
</head>
<body>
<input type="file" id="imageInput" accept="image/*">
<br>
<img id="previewImage" src="#" alt="预览图片" style="display: none; max-width: 100%; height: auto;">
<script>
document.getElementById('imageInput').addEventListener('change', function(event) {
const file = event.target.files[0];
if (file) {
const reader = new FileReader();
reader.onload = function(e) {
document.getElementById('previewImage').src = e.target.result;
document.getElementById('previewImage').style.display = 'block';
}
reader.readAsDataURL(file);
} else {
document.getElementById('previewImage').style.display = 'none';
}
});
</script>
</body>
</html>
```
### 后端实现
后端可以使用Spring Boot来实现图片上传接口。
```java
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.util.StringUtils;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.multipart.MultipartFile;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.UUID;
@RestController
@RequestMapping("/api")
public class ImageUploadController {
private static final String UPLOAD_DIR = "uploads/";
@PostMapping("/upload")
public ResponseEntity<?> uploadImage(@RequestParam("file") MultipartFile file) {
if (file.isEmpty()) {
return new ResponseEntity<>("文件为空", HttpStatus.BAD_REQUEST);
}
try {
// 创建目录
Path uploadPath = Paths.get(UPLOAD_DIR);
if (!Files.exists(uploadPath)) {
Files.createDirectories(uploadPath);
}
// 生成文件名
String fileName = StringUtils.cleanPath(file.getOriginalFilename());
String uniqueFileName = UUID.randomUUID().toString() + "_" + fileName;
// 保存文件
Path filePath = uploadPath.resolve(uniqueFileName);
Files.write(filePath, file.getBytes());
return new ResponseEntity<>("文件上传成功: " + uniqueFileName, HttpStatus.OK);
} catch (IOException e) {
return new ResponseEntity<>("文件上传失败", HttpStatus.INTERNAL_SERVER_ERROR);
}
}
}
```
### 说明
1. **前端**:使用HTML的`<input type="file">`标签来选择图片,并通过JavaScript的FileReader API读取图片文件并显示预览。
2. **后端**:使用Spring Boot的`@RestController`来处理文件上传请求,将上传的文件保存到服务器的指定目录。
通过这种方式,你可以实现一个简单的图片上传和预览功能。
阅读全文
相关推荐



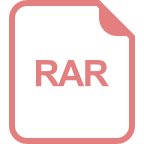













