用JavaScript实现计算每位学生的总分,并按总分排名输出学号和总分
时间: 2024-10-16 09:05:37 浏览: 48
在JavaScript中,我们可以创建一个简单的函数来实现这个功能。假设我们有一个学生数组,每个学生都有一个包含姓名和成绩的属性对象。以下是一个示例:
```javascript
// 假设学生数据结构如下
let students = [
{ id: 1, name: '张三', scores: [85, 90, 92] },
{ id: 2, name: '李四', scores: [78, 88, 95] },
// 更多学生...
];
function calculateTotalAndSort(students) {
// 计算每个学生的总分
students.forEach(student => {
student.totalScore = student.scores.reduce((sum, score) => sum + score, 0);
});
// 按总分排序
students.sort((a, b) => a.totalScore - b.totalScore);
// 输出学号和总分
students.forEach((student, index) => {
console.log(`${index + 1}. ${student.name}: 总分 ${student.totalScore}`);
});
}
calculateTotalAndSort(students);
相关问题
使用JavaScript语言 创建一个学生类(Student); 创建成绩集合(scores); 计算每个人的总分,并按总分排名输出学号和总分
在JavaScript中,我们可以创建一个名为`Student`的类来表示学生,包含姓名、学号以及成绩集合(scores)。下面是一个简单的例子:
```javascript
class Student {
constructor(name, id) {
this.name = name;
this.id = id;
this.scores = [];
}
addScore(score) {
this.scores.push(score);
}
calculateTotalScore() {
return this.scores.reduce((total, score) => total + score, 0);
}
}
// 示例学生对象
const student1 = new Student('张三', '001');
student1.addScore(85);
student1.addScore(90);
const student2 = new Student('李四', '002');
student2.addScore(92);
student2.addScore(95);
// 按总分排序并打印结果
students = [student1, student2];
students.sort((a, b) => b.calculateTotalScore() - a.calculateTotalScore());
students.forEach(student => {
console.log(`学号 ${student.id}, 总分 ${student.calculateTotalScore()}`);
});
```
在这个例子中,我们首先创建了一个`Student`类,然后创建了两个实例`student1`和`student2`,添加了一些成绩,并通过`calculateTotalScore`方法计算每个学生的总分。最后,我们将所有学生对象按照总分从高到低排序,然后打印出每位学生的学号和总分。
用JavaScript实现:以下是某个班级学生的成绩,分别包含学生的学号及其语文、数学、英语三科成绩,请按要求编写程序。 (1)计算每个人的总分,并按总分排名输出学号和总分。 (2)统计各单科成绩的前三名,并输出对应的学号和成绩。 关演示。 2 3 8 S 6 > 8 9 10 11 const scores =[ chinese:95,math:79,english:98 number:N10471 chinese:84,math:72,english: 76小 number:N1176, chinese:82,math: 99,english:97}, (number:N1087', chinese:77,math:89,english:701 {number:N18081 chinese:93,math;79,english:71 }. N1365 number: chinese:90,math: 91,english: 91 number:'N1416, chinese:74,math: 89,english: 85 ) number:'N1048 chinese: 74,math;82,english: 851 ( number:N1126 chinese:77,math:77,english: 85 1 { number:'N13861, { number:'N1869',chinese; 90,math; 74,english: 99}}
以下是用 JavaScript 实现您的要求的代码:
```javascript
const scores = [
{ number: 'N10471', chinese: 95, math: 79, english: 98 },
{ number: 'N1176', chinese: 84, math: 72, english: 76 },
{ number: 'N1087', chinese: 77, math: 89, english: 70 },
{ number: 'N18081', chinese: 93, math: 79, english: 71 },
{ number: 'N1365', chinese: 90, math: 91, english: 91 },
{ number: 'N1416', chinese: 74, math: 89, english: 85 },
{ number: 'N1048', chinese: 74, math: 82, english: 85 },
{ number: 'N1126', chinese: 77, math: 77, english: 85 },
{ number: 'N13861', chinese: 90, math: 74, english: 99 },
{ number: 'N1869', chinese: 90, math: 74, english: 99 },
];
// 计算每个人的总分,并按总分排名输出学号和总分
const totalScores = scores.map((student) => {
const total = student.chinese + student.math + student.english;
return { number: student.number, total };
}).sort((a, b) => b.total - a.total);
console.log('按总分排名输出学号和总分:');
totalScores.forEach((student, index) => {
console.log(`${index + 1}. 学号: ${student.number}, 总分: ${student.total}`);
});
// 统计各单科成绩的前三名,并输出对应的学号和成绩
const topChinese = scores.sort((a, b) => b.chinese - a.chinese).slice(0, 3);
console.log('语文前三名:');
topChinese.forEach((student) => {
console.log(`学号: ${student.number}, 成绩: ${student.chinese}`);
});
const topMath = scores.sort((a, b) => b.math - a.math).slice(0, 3);
console.log('数学前三名:');
topMath.forEach((student) => {
console.log(`学号: ${student.number}, 成绩: ${student.math}`);
});
const topEnglish = scores.sort((a, b) => b.english - a.english).slice(0, 3);
console.log('英语前三名:');
topEnglish.forEach((student) => {
console.log(`学号: ${student.number}, 成绩: ${student.english}`);
});
```
以上代码会先计算每个人的总分,并按总分排名输出学号和总分,然后统计各单科成绩的前三名,并输出对应的学号和成绩。
阅读全文
相关推荐
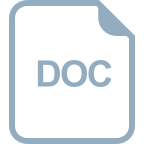
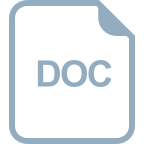
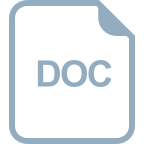
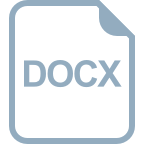
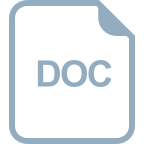
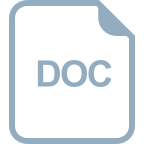










