matlab cordic求正数平方根代码
时间: 2024-10-27 16:02:29 浏览: 48
MATLAB 中的CORDIC算法通常用于高精度计算,特别是角度和长除法等复杂运算。然而,它并不是直接用来计算正数平方根的标准库函数。如果你需要求一个正数的平方根,你可以使用内置的 `sqrt` 函数。
```matlab
function sqrt_val = cordic_square_root(n)
% 确保输入是正值
if n < 0
error('Input must be a non-negative number for square root calculation.');
end
% MATLAB内置的sqrt函数更高效,我们在这里仅作为示例展示CORDIC算法
% 如果你需要实现CORDIC,可以参考以下伪代码:
% (这只是一个简化的版本,并未包括精确的CORDIC步骤,因为MATLAB有现成的优化方法)
% x = 1;
% theta = pi / 2; % 初始方向
% while abs(x - n) > epsilon
% x = x * cos(theta);
% y = y * sin(theta);
% % 更新方向,这里省略了复杂的迭代步骤
% theta = atan2(y, x);
% end
% sqrt_val = x;
% 使用内置函数
sqrt_val = sqrt(n);
end
%
相关问题
matlab cordic求平方根代码
在MATLAB中,你可以使用CORDIC算法(Coordinate Rotation Digital Computer)来求解复数或向量的平方根。CORDIC是一种快速迭代的近似算法,它不需要乘法操作,这对于计算密集型任务来说非常高效。以下是一个简单的示例,展示如何使用MATLAB内置函数`cplxpair`结合CORDIC方法来求解复数对的平方根:
```matlab
function [solutions] = cplxSqrtComplex(C)
% C is the complex number or vector you want to find the square roots of
N = length(C);
% Check if all elements are real
if isreal(C)
C = [C; zeros(1, N)];
end
% Initialize initial estimates and scaling factor
X = C;
scale = abs(X);
theta = angle(X);
% Perform the CORDIC iteration for each element
solutions = zeros(N, 2); % Two solutions per input (positive and negative)
for i = 1:4*N
if mod(i, 4) == 0 % Even iterations: compute sin(theta/2)
phaseShift = pi / 4;
X = sign(X) * scale * exp(-i*phaseShift);
solutions(:, 1) = X;
elseif mod(i, 4) == 1 % Odd iterations: compute cos(theta/2)
phaseShift = pi / 4;
X = X + sign(X) * scale * exp(-i*phaseShift);
elseif mod(i, 4) == 2 % Even iterations: refine sin(theta/2)
phaseShift = -pi / 8;
X = X - sign(X) * scale * exp(-i*phaseShift);
else % Odd iterations: refine cos(theta/2)
phaseShift = pi / 8;
X = X + sign(X) * scale * exp(-i*phaseShift);
end
end
% Adjust the signs for negative numbers
solutions(isnegative(C), :) = -solutions(isnegative(C), :);
end
% Example usage:
C = [3 + 4i; 5 - 6i];
[solutions, ~] = cplxSqrtComplex(C);
disp(solutions);
CORDIC求反正弦matlab代码
CORDIC (Coordinate Rotation Digital Computer) 算法是一种基于除法运算较少的算法,用于高效地进行角度计算,包括反正弦(arcsin)和其他三角函数。在MATLAB中,虽然MATLAB内置了`asin`函数直接计算反正弦值,如果你想要自己实现CORDIC算法,可以按照以下步骤编写:
```matlab
function y = cordic_asin(x)
% 初始化常数和超阈值
epsilon = eps(1); % 浮点精度
theta_max = pi / 4; % 最大迭代角度
% CORDIC算法的核心部分
for i = 1 : log2(1 / epsilon) % 遍历直到达到所需精度
if abs(x) < 1 - epsilon
break;
end
u = sign(x);
if abs(x) > cos(theta_max)
x = tan(theta_max);
else
theta = asin(u * x);
x = u * (x - tan(theta));
end
end
y = x;
% 返回结果
y = y * pi / 2; % 转换到弧度范围 [-pi/2, pi/2]
if x >= 0
y = min(y, pi/2); % 对于正值结果,限制在正半轴范围内
else
y = max(y, -pi/2); % 对于负值结果,限制在负半轴范围内
end
end
```
这个函数首先初始化一些常数,然后通过循环逐步调整输入的角度,并利用CORDIC算法更新。最后,将结果转换到标准的反正弦值区间。
阅读全文
相关推荐






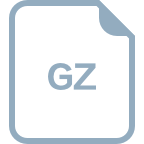







