大一c语言餐馆点菜系统运用结构体和链表
时间: 2024-08-28 07:02:43 浏览: 61
一个基于C语言的餐馆点菜系统可以利用结构体和链表来实现。在这样的系统中,结构体可以用来定义菜品和订单的信息,而链表则用来管理这些信息,使系统能够处理多个菜品和订单。
首先,我们定义一个菜品的结构体,它可能包含菜品名称、价格、数量等信息。例如:
```c
typedef struct Dish {
char name[50]; // 菜品名称
float price; // 菜品价格
int quantity; // 菜品数量
struct Dish *next; // 指向下一个菜品的指针
} Dish;
```
接着,我们可以通过链表将多个菜品连接起来,形成一个菜品的菜单。同样,我们也可以为顾客的订单创建一个类似的结构体和链表结构。
在点菜系统中,我们可能需要执行以下操作:
1. 添加菜品到菜单。
2. 允许顾客根据菜单添加菜品到他们的订单。
3. 更新订单中的菜品数量和价格。
4. 计算订单总价。
链表提供了动态数据结构的优点,比如可以随时添加或删除节点,而不需要事先知道数据的总量。这样,即使在运行时,顾客点的菜品数量增加或减少,系统也能够灵活应对。
例如,如果顾客想要添加一个新菜品到订单中,我们可以创建一个新的Dish结构体实例,并将其插入到订单链表的相应位置。如果顾客想要修改某个菜品的数量,我们可以在链表中找到对应的节点,并更新其数量和总价。
系统最终会计算所有订单项的总价,并可能打印出一个顾客的点菜单,包括所有菜品的名称、数量和价格。
相关问题
运用结构体和链表小餐馆点餐系统c语言完整代码
在C语言中,要实现一个简单的小餐馆点餐系统,我们可以使用结构体来存储菜品信息,包括菜品名称、价格等。同时,我们可以使用链表来管理这些结构体变量,因为链表可以动态地增加或删除节点,非常适合处理动态的数据集合。
下面是一个简单的示例代码,展示了如何定义结构体、创建链表以及实现一个简单的点餐系统。请注意,这个示例是为了演示目的,可能不包含一个完整系统所需的所有功能。
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 定义菜品结构体
typedef struct Dish {
char name[30];
float price;
struct Dish *next;
} Dish;
// 创建新节点
Dish* createDish(const char* name, float price) {
Dish* newDish = (Dish*)malloc(sizeof(Dish));
if (newDish == NULL) {
fprintf(stderr, "内存分配失败\n");
exit(1);
}
strcpy(newDish->name, name);
newDish->price = price;
newDish->next = NULL;
return newDish;
}
// 向链表中添加菜品
void addDish(Dish **head, const char* name, float price) {
Dish *newDish = createDish(name, price);
if (*head == NULL) {
*head = newDish;
} else {
Dish *current = *head;
while (current->next != NULL) {
current = current->next;
}
current->next = newDish;
}
}
// 打印菜单
void printMenu(Dish *head) {
printf("菜单:\n");
Dish *current = head;
while (current != NULL) {
printf("%s - ¥%.2f\n", current->name, current->price);
current = current->next;
}
}
// 点餐
void orderDish(Dish *head, const char* name) {
Dish *current = head;
while (current != NULL) {
if (strcmp(current->name, name) == 0) {
printf("已点:%s - ¥%.2f\n", current->name, current->price);
return;
}
current = current->next;
}
printf("菜单上没有找到名为 %s 的菜品。\n", name);
}
// 主函数
int main() {
Dish *menu = NULL; // 创建空链表作为菜单
// 添加菜品到菜单
addDish(&menu, "宫保鸡丁", 32.0);
addDish(&menu, "鱼香肉丝", 28.0);
addDish(&menu, "青椒肉丝", 26.0);
// 打印菜单
printMenu(menu);
// 点餐
orderDish(menu, "宫保鸡丁");
orderDish(menu, "青椒土豆丝"); // 假设菜单上没有这道菜
// 清理工作:删除链表
Dish *current = menu;
while (current != NULL) {
Dish *next = current->next;
free(current);
current = next;
}
return 0;
}
```
在这个例子中,我们定义了一个`Dish`结构体来存储菜品的信息,并创建了一个链表来管理这些菜品。我们添加了几个辅助函数来处理创建新菜品、添加菜品到菜单、打印菜单和点菜的功能。
阅读全文
相关推荐
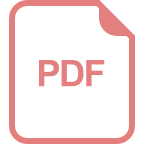
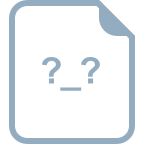
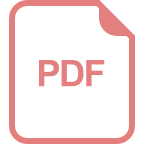
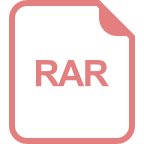
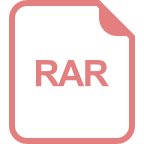
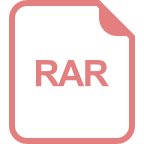
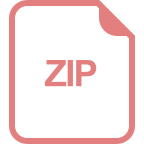
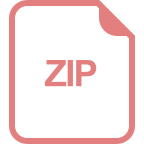
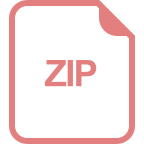
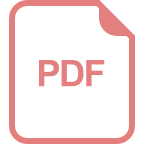
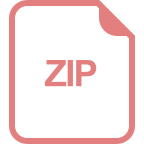
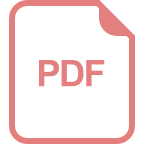
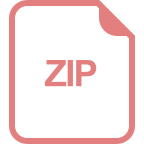