首先分别实现顺序栈、链栈的各种基本运算;其次设计程序调用顺序栈、链栈的这些算法进行功能测试。使用c++,并给出完整代码
时间: 2024-11-30 10:18:19 浏览: 2
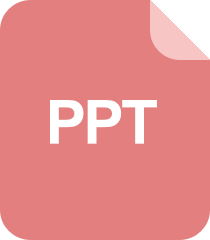
在顺序栈中实现栈的基本运算算法:-数据结构讲义
首先,我们来创建顺序栈(Array Stack)和链栈(Linked List Stack)的基本运算。以下是使用C++实现它们以及进行功能测试的一个例子:
**顺序栈(Array Stack)实现:**
```cpp
#include <iostream>
using namespace std;
#define MAX_SIZE 100
class ArrayStack {
private:
int top;
int data[MAX_SIZE];
public:
ArrayStack() : top(-1) {}
bool isEmpty() { return top == -1; }
bool isFull() { return top == MAX_SIZE - 1; }
void push(int value) {
if (isFull()) {
cout << "Stack overflow.\n";
return;
}
top++;
data[top] = value;
}
int pop() {
if (isEmpty()) {
cout << "Stack underflow.\n";
return -1;
}
int value = data[top];
top--;
return value;
}
int peek() const {
if (isEmpty()) {
cout << "Stack is empty.\n";
return -1;
}
return data[top];
}
// 功能测试
int main() {
ArrayStack arrStack;
arrStack.push(1);
arrStack.push(2);
cout << "Top element: " << arrStack.peek() << endl;
cout << "Popped element: " << arrStack.pop() << endl;
return 0;
}
```
**链栈(Linked List Stack)实现:**
```cpp
#include <iostream>
struct Node {
int data;
Node* next;
};
class LinkedListStack {
private:
Node* top;
public:
LinkedListStack() : top(nullptr) {}
bool isEmpty() { return top == nullptr; }
void push(int value) {
Node* newNode = new Node();
newNode->data = value;
newNode->next = top;
top = newNode;
}
int pop() {
if (isEmpty()) {
cout << "Stack underflow.\n";
return -1;
}
int value = top->data;
Node* temp = top;
top = top->next;
delete temp;
return value;
}
int peek() const {
if (isEmpty()) {
cout << "Stack is empty.\n";
return -1;
}
return top->data;
}
// 其他辅助函数...
};
// 功能测试
int main() {
LinkedListStack lnkStack;
lnkStack.push(1);
lnkStack.push(2);
cout << "Top element: " << lnkStack.peek() << endl;
cout << "Popped element: " << lnkStack.pop() << endl;
return 0;
}
```
阅读全文
相关推荐
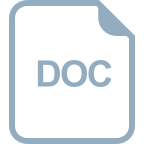
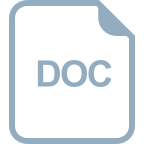
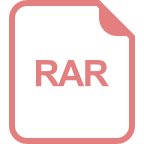
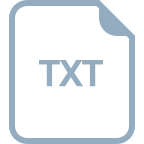
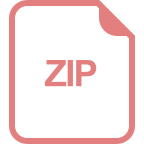
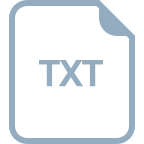
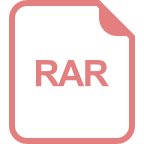
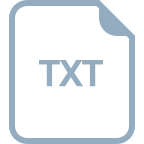
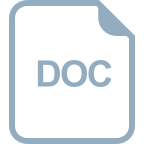
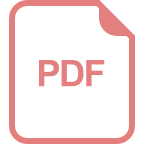
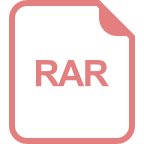
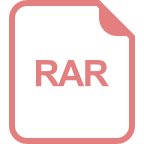
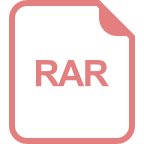
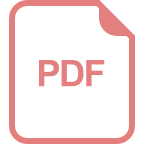
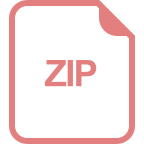
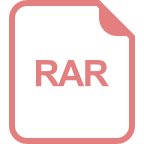
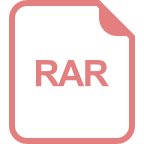
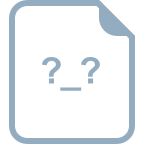