编写一个程序,创建一个有名管道,并使用两个进程,一个进程从一个文件中读取一些字符串,并将其写入到管道中,另一个进程从管道中读取字符串并统计其中的字符数并输出。
时间: 2024-09-30 13:12:08 浏览: 67
在Unix/Linux系统中,可以使用有名管道(named pipe)或匿名管道(pipe)来实现在两个进程之间共享数据。下面是一个使用有名管道的C程序示例,展示了如何读取文件内容并将字符串写入管道,以及从管道读取字符串并计算字符数的过程。
首先,创建一个名为`data.pipe`的有名管道:
```bash
mkfifo data.pipe
```
然后编写主程序,分为读写两个部分:
**reader.c**
```c
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
#define BUFFER_SIZE 1024
int main() {
int pipe_fd[2], read_count = 0;
char buffer[BUFFER_SIZE];
if (pipe(pipe_fd) < 0) {
perror("Pipe creation failed");
return 1;
}
close(pipe_fd[1]); // 只读端,关闭写端
FILE *file = fopen("input.txt", "r");
if (!file) {
perror("Opening file failed");
return 1;
}
while (fgets(buffer, sizeof(buffer), file)) {
write(pipe_fd[0], buffer, strlen(buffer));
read_count += strlen(buffer);
}
fclose(file);
close(pipe_fd[0]);
printf("Read %d characters from file and wrote them to the pipe.\n", read_count);
return 0;
}
```
**writer.c**
```c
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
int main() {
int pipe_fd[2];
char buffer[1024];
ssize_t bytes_read;
if (pipe(pipe_fd) < 0) {
perror("Pipe creation failed");
return 1;
}
close(pipe_fd[0]); // 只写端,关闭读端
while ((bytes_read = read(pipe_fd[1], buffer, sizeof(buffer))) > 0) {
buffer[bytes_read] = '\0'; // 结束字符串
printf("Read: %s, Count: %lu\n", buffer, (unsigned long)strcspn(buffer, "\n"));
}
if (bytes_read == -1) {
perror("Reading from pipe failed");
}
close(pipe_fd[1]);
return 0;
}
```
运行流程:
1. 创建`writer.c`进程,它打开`input.txt`文件并逐行读取,将每行字符串写入管道。
2. 创建`reader.c`进程,它连接到管道,读取字符串并统计字符数。
3. `writer.c`进程完成后,`reader.c`进程开始统计并输出字符总数。
注意,你需要编译这两个程序并以不同的进程启动它们,因为它们都在尝试打开同一管道。同时,这里假设`input.txt`文件存在并且有可用的数据。
阅读全文
相关推荐
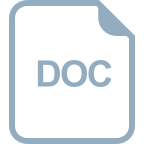
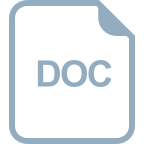
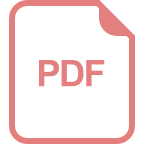
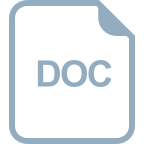
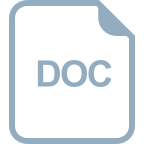
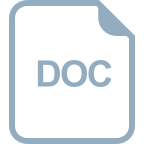
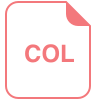
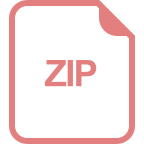
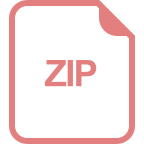
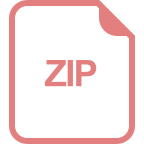
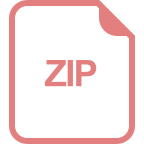
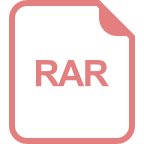
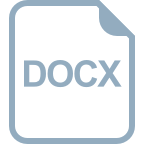
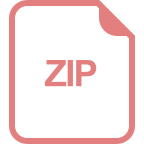
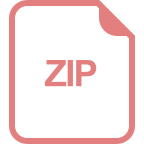