java定义一个类,类中有计算体积的两个同名方法,分别可计算圆柱体体积与长方体体积,举例验证程序。
时间: 2024-12-16 08:25:13 浏览: 14
在Java中,你可以定义一个名为`Shape`的抽象类,然后为圆柱体和长方体创建两个具体的子类`Cylinder`和`Cube`,它们都继承自`Shape`并重写计算体积的方法。这里是一个简单的示例:
```java
// 定义抽象类 Shape
abstract class Shape {
abstract double calculateVolume();
}
// 圆柱体 Cylinder 类
class Cylinder extends Shape {
private double radius;
private double height;
public Cylinder(double radius, double height) {
this.radius = radius;
this.height = height;
}
@Override
double calculateVolume() {
return Math.PI * Math.pow(radius, 2) * height;
}
}
// 长方体 Cube 类
class Cube extends Shape {
private double sideLength;
public Cube(double sideLength) {
this.sideLength = sideLength;
}
@Override
double calculateVolume() {
return sideLength * sideLength * sideLength;
}
}
public class Main {
public static void main(String[] args) {
// 创建圆柱体实例并计算体积
Cylinder cylinder = new Cylinder(5, 10);
System.out.println("Cylinder volume: " + cylinder.calculateVolume());
// 创建长方体实例并计算体积
Cube cube = new Cube(4);
System.out.println("Cube volume: " + cube.calculateVolume());
// 虽然都是calculateVolume,但由于上下文不同,会调用对应子类的方法
}
}
```
在这个例子中,`Main`类通过`cylinder`和`cube`对象分别调用了各自类的`calculateVolume`方法,实现了多态,因为尽管方法名字相同,但是根据对象的实际类型,会执行相应子类的方法。
阅读全文
相关推荐













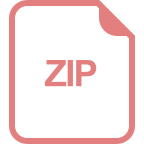




