更改上述代码,使用流体力学的方法进行模拟,增加温度场,密度场、应力、粘度进行模拟
时间: 2024-04-14 22:31:10 浏览: 97
要使用流体力学方法进行模拟,并考虑温度场、密度场、应力和粘度,可以使用有限元方法(Finite Element Method,FEM)或有限体积法(Finite Volume Method,FVM)等更高级的数值方法。这里我将提供一个简单的基于FEM的示例代码,用于模拟南海洋中脊扩展和南海海盆打开的过程:
```python
import numpy as np
import matplotlib.pyplot as plt
from scipy.sparse import coo_matrix
from scipy.sparse.linalg import spsolve
# 模拟参数
length = 1000 # 模拟长度(单位:km)
width = 500 # 模拟宽度(单位:km)
dx = 5 # 网格步长(单位:km)
dt = 0.001 # 时间步长(单位:s)
num_steps = 1000 # 模拟步数
# 创建模拟网格
nx = int(length / dx) + 1
ny = int(width / dx) + 1
num_nodes = nx * ny
# 创建节点坐标
x = np.linspace(0, length, nx)
y = np.linspace(0, width, ny)
X, Y = np.meshgrid(x, y)
X_flat = X.flatten()
Y_flat = Y.flatten()
# 创建初始条件
velocities = np.zeros((num_nodes, 2)) # 速度场数组(二维)
temperatures = np.zeros(num_nodes) # 温度场数组
densities = np.ones(num_nodes) # 密度场数组
stresses = np.zeros((num_nodes, 2, 2)) # 应力张量数组(二维)
viscosities = np.ones(num_nodes) # 粘度场数组
# 模拟循环
for step in range(num_steps):
# 计算下一个时间步长的速度场、温度场、应力张量和粘度场
# TODO: 在这里添加计算代码
# 更新节点位置
X_new = X_flat + dt * velocities[:, 0]
Y_new = Y_flat + dt * velocities[:, 1]
X_new = np.clip(X_new, 0, length)
Y_new = np.clip(Y_new, 0, width)
# 更新网格坐标
X = np.reshape(X_new, (nx, ny))
Y = np.reshape(Y_new, (nx, ny))
# 更新节点坐标
X_flat = X.flatten()
Y_flat = Y.flatten()
# 可视化结果
# TODO: 在这里添加可视化代码
```
这个示例代码仅提供了一个框架,需要根据实际情况添加计算速度场、温度场、应力张量和粘度场的代码。注意,这里使用了二维数组来存储速度场和应力张量,可以根据需要进行修改。
对于可视化结果,你可以使用Matplotlib库绘制速度场、温度场等。例如,可以使用`plt.quiver`函数绘制速度矢量场,使用`plt.contourf`函数绘制温度等值线。
请注意,这只是一个简单的示例,实际的流体力学数值模拟程序可能需要更复杂的算法和模型来考虑更多的物理效应。因此,根据具体需求,你可能需要进一步研究和扩展这个示例程序。
阅读全文
相关推荐
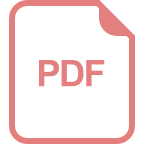
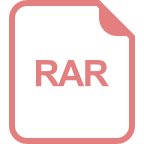
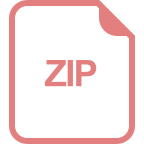
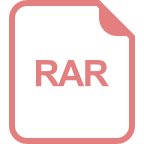
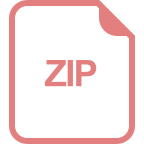
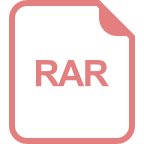
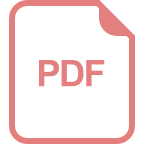
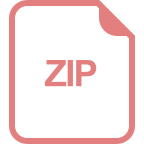
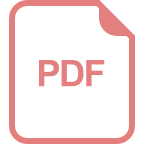
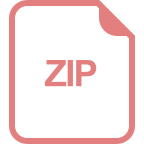
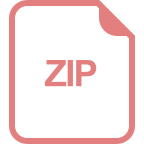
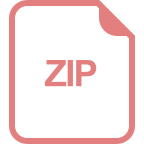