BFS迷宫问题python
时间: 2023-12-01 11:43:45 浏览: 54
以下是使用BFS算法解决迷宫问题的Python代码:
```python
from queue import Queue
# 定义迷宫地图
maze = [
[0, 1, 0, 0, 0],
[0, 1, 0, 1, 0],
[0, 0, 0, 0, 0],
[0, 1, 1, 1, 0],
[0, 0, 0, 1, 0]
]
# 定义起点和终点
start = (0, 0)
end = (4, 4)
# 定义四个方向
directions = [(0, 1), (0, -1), (1, 0), (-1, 0)]
# 定义BFS算法
def BFS(maze, start, end):
queue = Queue()
queue.put(start)
visited = set()
visited.add(start)
while not queue.empty():
cur_x, cur_y = queue.get()
if cur_x == end[0] and cur_y == end[1]:
return True
for direction in directions:
new_x = cur_x + direction[0]
new_y = cur_y + direction[1]
if 0 <= new_x < len(maze) and 0 <= new_y < len(maze[0]) and maze[new_x][new_y] == 0 and (new_x, new_y) not in visited:
queue.put((new_x, new_y))
visited.add((new_x, new_y))
return False
# 调用BFS算法
print(BFS(maze, start, end))
```
该代码使用队列实现BFS算法,从起点开始遍历迷宫地图,直到找到终点或者遍历完整个地图。在遍历过程中,使用visited集合记录已经遍历过的点,避免重复遍历。如果找到终点,返回True,否则返回False。
相关推荐
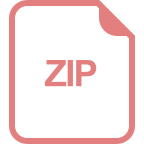
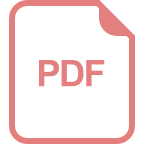
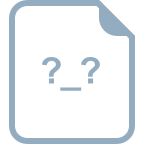














