设链表结点结构已经给出,试用c语言编写链表插入函数Insert将实现链表末尾添加数据,再编写函数Print,实现链表中链表数据打印,编写Destroy函数,实现链表的销毁释放,并在main函数中写出完整的调用过程。
时间: 2023-07-11 07:30:31 浏览: 124
链表结点结构如下:
```c
typedef struct Node{
int data;
struct Node *next;
}Node, *LinkList;
```
链表插入函数Insert的实现:
```c
void Insert(LinkList *L, int data){
Node *p = (Node*)malloc(sizeof(Node));
p->data = data;
p->next = NULL;
if(*L == NULL){
*L = p;
return;
}
Node *q = *L;
while(q->next != NULL){
q = q->next;
}
q->next = p;
}
```
链表打印函数Print的实现:
```c
void Print(LinkList L){
if(L == NULL){
printf("链表为空");
return;
}
Node *p = L;
while(p){
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
```
链表销毁函数Destroy的实现:
```c
void Destroy(LinkList *L){
Node *p = *L;
while(p){
Node *q = p;
p = p->next;
free(q);
}
*L = NULL;
}
```
在main函数中的调用过程:
```c
int main(){
LinkList L = NULL;
Insert(&L, 1);
Insert(&L, 2);
Insert(&L, 3);
Print(L);
Destroy(&L);
return 0;
}
```
输出结果为:1 2 3
阅读全文
相关推荐





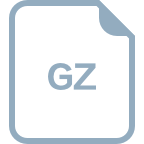



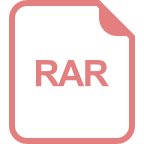
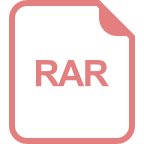


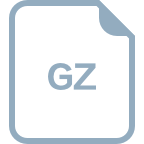

