自定义停用词表去除文件夹下所有文件的中文停用词并写入新文件,python代码
时间: 2024-05-14 11:19:04 浏览: 10
以下是用Python实现自定义停用词表去除文件夹下所有文件的中文停用词并写入新文件的代码:
```python
import os
import jieba
# 定义停用词表路径
stopwords_path = 'stopwords.txt'
# 加载停用词表
stopwords = []
with open(stopwords_path, 'r', encoding='utf-8') as f:
for line in f:
stopwords.append(line.strip())
# 定义文件夹路径
folder_path = '/path/to/folder'
# 遍历文件夹下所有文件
for root, dirs, files in os.walk(folder_path):
for file in files:
file_path = os.path.join(root, file)
with open(file_path, 'r', encoding='utf-8') as f:
content = f.read()
# 使用 jieba 分词
words = jieba.cut(content)
# 去除停用词
words = [word for word in words if word not in stopwords]
# 将处理后的文本写入新文件
new_file_path = file_path.replace('.txt', '_without_stopwords.txt')
with open(new_file_path, 'w', encoding='utf-8') as f:
f.write(' '.join(words))
```
在上面的代码中,我们首先加载了自定义的停用词表,然后使用 `os.walk` 遍历了指定文件夹下的所有文件。对于每个文件,我们使用 `jieba` 进行中文分词,并去除停用词,最终将处理后的文本写入一个新文件中。需要注意的是,我们使用了 `replace` 方法将原文件名中的 `.txt` 替换为 `_without_stopwords.txt`,以便区分处理前后的文件。
相关推荐
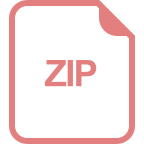
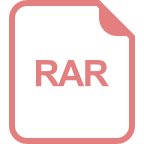
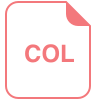













