用python设计一个表示动物(Animal)的类,该类包括颜色(color)属性和叫(call)方法。再设计一个表示鱼(Fish)的类,包括尾巴(tail)和颜色(color)两个属性,及叫(call)方法。
时间: 2024-05-15 11:17:41 浏览: 72
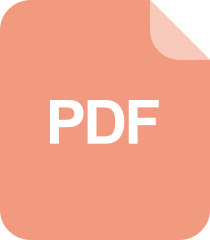
python中类的属性和方法介绍
好的,下面是用 Python 设计表示动物和鱼的类:
```python
# 表示动物的类 Animal
class Animal:
def __init__(self, color):
self.color = color
def call(self):
print("叫声......")
# 表示鱼的类 Fish
class Fish:
def __init__(self, tail, color):
self.tail = tail
self.color = color
def call(self):
print("鱼儿不会叫,只能水中游......")
```
以上代码实现了一个基本的动物类和鱼类,它们都包括颜色属性和叫方法,但鱼类多了一个尾巴属性。当调用它们的叫方法时,动物会有叫声,而鱼只能在水中游。你可以根据自己的需要对它们进行扩展和修改。
阅读全文
相关推荐







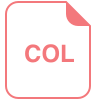
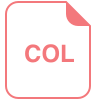
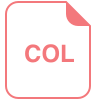
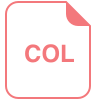
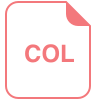
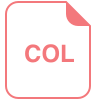
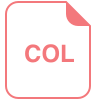
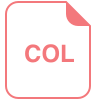
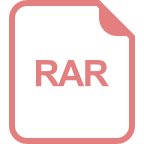
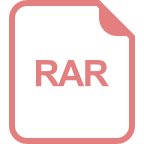