在pyspark mllib上,实现SVM对于新闻的分类。数据集为多个按新闻类型分类的文件夹,每个文件夹下为对应类别的新闻正文文本。如何通过sparkcontext获取数据集,数据集存放在hdfs上,转换成RDD形式。要求对正文的数据集进行tf-idf处理,数据清洗,停用词处理等。同时注意中文的乱码问题,文本为ans
时间: 2024-01-21 15:16:40 浏览: 212
首先需要导入必要的库和模块:
```python
from pyspark import SparkContext
from pyspark.sql import SQLContext
from pyspark.ml.feature import HashingTF, IDF
from pyspark.ml.classification import LinearSVC
from pyspark.ml import Pipeline
from pyspark.ml.feature import Tokenizer, RegexTokenizer, StopWordsRemover
import jieba
import re
```
然后我们可以创建一个SparkContext对象,并从HDFS中读取数据集:
```python
sc = SparkContext(appName="SVM_classification")
sqlContext = SQLContext(sc)
# 从HDFS中读取数据集
data = sqlContext.read.text("hdfs://path/to/dataset/*/*").rdd
```
接下来,我们需要对数据进行预处理。首先,我们需要将每个新闻正文进行分词,并去掉停用词:
```python
# 定义一个函数进行分词和去停用词
def segment_words(line):
# 加载自定义的停用词表
stopwords = set(open("stopwords.txt", "r", encoding="utf-8").read().splitlines())
# 对每行数据进行分词
words = jieba.cut(line.strip(), cut_all=False)
# 去掉停用词
return [word for word in words if word not in stopwords]
# 对数据集中的每一行进行分词和去停用词
segmented_data = data.map(lambda x: (x[0], segment_words(x[0])))
```
然后,我们需要将分词后的文本转换成向量形式,以便进行后续的机器学习模型训练。我们可以使用HashingTF和IDF来实现这一步骤:
```python
# 定义HashingTF和IDF对象
hashingTF = HashingTF(inputCol="words", outputCol="rawFeatures", numFeatures=10000)
idf = IDF(inputCol="rawFeatures", outputCol="features")
# 构建Pipeline对象来对数据进行处理
pipeline = Pipeline(stages=[hashingTF, idf])
# 对数据集中的每一行进行处理
pipeline_model = pipeline.fit(segmented_data)
processed_data = pipeline_model.transform(segmented_data)
```
最后,我们可以使用LinearSVC模型来训练我们的分类器,并对测试数据进行预测:
```python
# 将数据集划分为训练集和测试集
(training_data, test_data) = processed_data.randomSplit([0.8, 0.2], seed=1234)
# 定义LinearSVC模型
svm = LinearSVC(maxIter=10, regParam=0.1)
# 训练模型
svm_model = svm.fit(training_data)
# 对测试数据进行预测
predictions = svm_model.transform(test_data)
```
至此,我们就完成了对于新闻文本的分类任务。
阅读全文
相关推荐
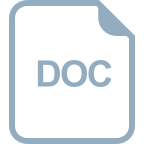
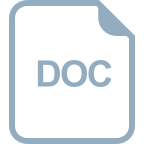
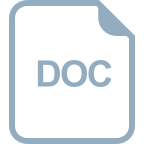
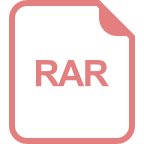
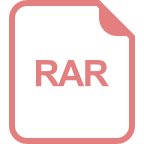
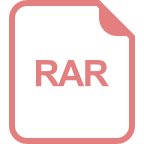
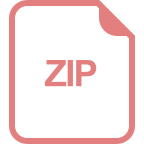
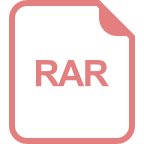
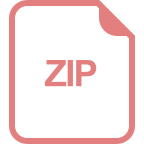
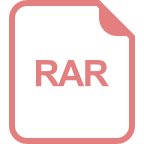
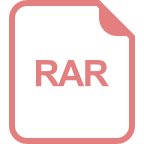
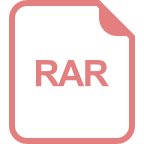
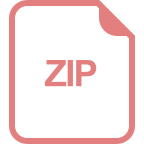
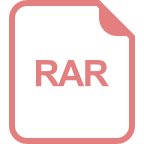
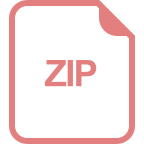
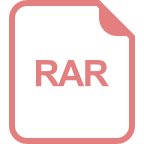
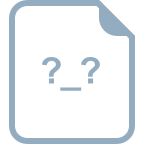
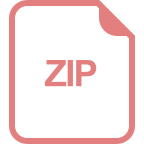