tensorflow.python.framework.errors_impl.InvalidArgumentError: cannot compute MatMul as input #1(zero-based) was expected to be a double tensor but is a float tensor [Op:MatMul]
时间: 2024-02-18 14:59:04 浏览: 177
这个错误是由于您的代码中使用了双精度张量和浮点数张量进行矩阵乘法运算,导致 TensorFlow 无法计算。在 TensorFlow 中,双精度张量和浮点数张量是不兼容的,因此在进行矩阵乘法运算之前,需要将它们转换为相同的数据类型。
为了解决这个错误,您可以通过将张量转换为双精度或浮点数来使它们具有相同的数据类型。例如,如果您的输入张量是浮点数类型,您可以使用 `tf.cast` 函数将其转换为双精度类型:
```python
import tensorflow as tf
input_float = tf.Variable([[1.0, 2.0], [3.0, 4.0]])
input_double = tf.cast(input_float, tf.float64) # 将浮点数张量转换为双精度张量
result = tf.matmul(input_double, input_double) # 执行矩阵乘法运算
```
在上面的示例代码中,我们首先定义了一个浮点数张量 `input_float`,然后使用 `tf.cast` 函数将其转换为双精度张量 `input_double`。最后,我们使用 `tf.matmul` 函数执行矩阵乘法运算。
如果您的输入张量是双精度类型,则可以使用类似的方法将其转换为浮点数类型。
相关问题
tensorflow.python.framework.errors_impl.invalidargumenterror:
### 回答1:
tensorflow.python.framework.errors_impl.invalidargumenterror是TensorFlow框架中的一个错误类型,表示传递给函数的参数无效。可能是参数类型不匹配,参数值超出了允许的范围,或者参数缺失等。需要检查参数是否正确,并进行相应的修正。
### 回答2:
TensorFlow是一个非常受欢迎的深度学习框架,它被广泛应用于各种计算机视觉、自然语言处理、推荐系统和强化学习任务中。然而在使用TensorFlow的过程中,我们可能遇到各种各样的错误。其中,tensorflow.python.framework.errors_impl.invalidargumenterror是一种常见的错误类型。
tensorflow.python.framework.errors_impl.invalidargumenterror错误通常会在TensorFlow的计算图中的一个操作出现了无效的参数或不合法的输入数据时发生。这种错误可以来源于多个方面,比如:
1. 参数维度不匹配:当我们调用某个TensorFlow操作时,如果传入的tensor与该操作要求的shape不符,就会发生这种错误。这时候需要检查输入数据的维度是否正确,是否需要进行reshape等操作。
2. 数据类型错误:有些TensorFlow操作只能接受特定类型的数据作为输入,如果我们传入了错误类型的数据,就会产生这种错误。这时候需要检查数据类型是否正确,是否需要进行类型转换等操作。
3. 无效的张量:如果我们传递了一个无效的tensor作为某个操作的输入,就会发生这种错误。这种情况下需要检查输入的张量是否已经被正确创建,是否需要重新创建该张量以消除错误。
4. 数据范围错误:有些TensorFlow操作要求输入数据在一定范围内,如果我们输入的数据超出了该范围,就会发生这种错误。这时候需要检查输入数据是否合法,是否需要进行数据归一化等操作。
针对以上情况,我们可以通过以下措施进行解决:
1. 仔细检查代码:当出现tensorflow.python.framework.errors_impl.invalidargumenterror错误时,我们应该首先仔细检查代码,查找可能导致错误的操作。
2. 进行数据调试:如果发现数据维度不匹配或数据类型错误等问题,我们可以通过打印相关变量的shape和dtype信息以及使用TensorBoard查看计算图的方式来调试数据。
3. 重建张量:如果发现输入张量无效,可以通过重新创建张量的方式进行修复。
4. 限定数据范围:如果发现数据范围错误,可以对数据进行归一化、截断等操作。
综上所述,tensorflow.python.framework.errors_impl.invalidargumenterror是一种常见的TensorFlow错误类型,出现该错误时需要仔细排查代码和数据,并根据具体情况进行相应的处理。
### 回答3:
tensorflow.python.framework.errors_impl.invalidargumenterror: 是TensorFlow的一个错误类型,通常表示某些参数不符合要求或不合法。
其可能产生的原因有:
1.输入数据的形状不正确:在使用张量进行计算时,如果张量的形状不符合要求,就会出现这种错误。例如,如果某个操作要求输入数据形状为(batch_size,width,height,channel),但输入的数据形状为(height,width,channel),就会报错。
2. 参数设置不当:在使用某些函数或操作时,需要传入一些参数,如果这些参数不合法,也容易引起这种错误。例如,卷积操作的步长参数必须是正整数,如果传入了负数或零,就会报错。
3. 数据类型不匹配:在使用某些操作时,可能需要传入特定的数据类型,如果传入的数据类型与要求不符,就会报错。例如,某一层要求输入数据类型为float32,但传入了int类型的数据,就会报错。
4. GPU内存不足:如果在使用GPU时,内存不足,也会出现这种错误。这时可以尝试减小batch大小或使用更小的模型来减少内存占用。
解决这种错误的方法通常是分析错误信息,找出导致错误的原因,并修正代码或参数设置。同时可以查阅TensorFlow文档或社区,寻找解决方法。
tensorflow.python.framework.errors_impl.InvalidArgumentError: required broadcastable shapes [Op:Sub]
This error occurs when the shape of the input tensors in a subtraction operation cannot be broadcasted. Broadcasting is the process of making the shapes of the input tensors compatible for performing element-wise operations such as addition or subtraction.
To resolve this error, you need to check the shapes of the input tensors and make sure they are compatible for broadcasting. The shapes of the tensors must match or be compatible according to the rules of broadcasting.
For example, if you are subtracting two tensors A and B, their shapes must satisfy one of the following conditions:
- A and B have the same shape.
- One of the dimensions of A or B is 1, and the other dimension is the same for both tensors.
- One of the dimensions of A or B is missing (i.e., None), and the other dimension is the same for both tensors.
If the shapes of the input tensors are not compatible for broadcasting, you may need to reshape or transpose them to make them compatible. You can use the tf.reshape and tf.transpose functions in TensorFlow to manipulate the shapes of tensors.
阅读全文
相关推荐
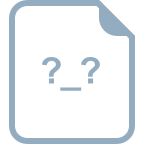
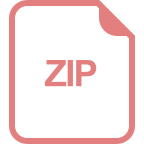
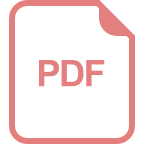

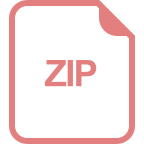
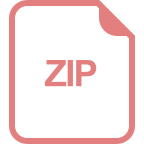
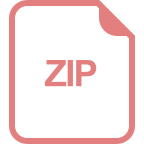
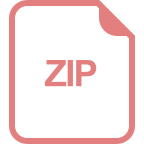
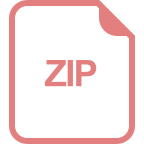
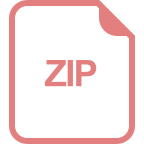
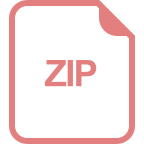
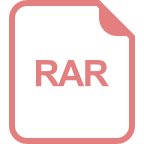
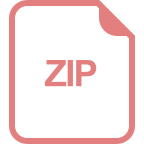
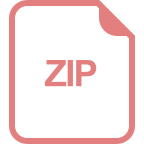
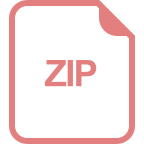