编写程序统计一个输入的Java源码中关键字(区分大小写)出现的次数。说明如下: Java中共有53个关键字(自行百度) 从键盘输入一段源码,统计这段源码中出现的关键字的数量 注释中出现的关键字不用统计 字符串中出现的关键字不用统计 统计出的关键字及数量按照关键字升序进行排序输出 未输入源码则认为输入非法 输入格式: 输入Java源码字符串,可以一行或多行,以exit行作为结束标志 输出格式: 当未输入源码时,程序输出Wrong Format 当没有统计数据时,输出为空 当有统计数据时,关键字按照升序排列,每行输出一个关键字及数量,格式为数量\t关键字,java实现
时间: 2024-03-14 11:42:59 浏览: 65
下面是一个示例Java程序,用于统计输入的Java源码中出现的关键字的数量,并按照关键字升序进行排序输出:
```java
import java.util.*;
public class KeywordCounter {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
String line;
StringBuilder builder = new StringBuilder();
while (!(line = scanner.nextLine()).equals("exit")) {
builder.append(line);
builder.append("\n");
}
String sourceCode = builder.toString();
if (sourceCode.trim().isEmpty()) {
System.out.println("Wrong Format");
return;
}
String[] keywords = new String[] {
"abstract", "assert", "boolean", "break", "byte", "case", "catch", "char", "class", "const",
"continue", "default", "do", "double", "else", "enum", "extends", "final", "finally", "float",
"for", "goto", "if", "implements", "import", "instanceof", "int", "interface", "long", "native",
"new", "package", "private", "protected", "public", "return", "short", "static", "strictfp",
"super", "switch", "synchronized", "this", "throw", "throws", "transient", "try", "void",
"volatile", "while"
};
Map<String, Integer> keywordCountMap = new TreeMap<>();
int startPos = 0;
boolean inString = false;
boolean inComment = false;
while (startPos < sourceCode.length()) {
int nextPos;
if (inString) {
nextPos = sourceCode.indexOf("\"", startPos + 1);
if (nextPos == -1) {
break;
}
} else if (inComment) {
nextPos = sourceCode.indexOf("*/", startPos + 1);
if (nextPos == -1) {
break;
}
inComment = false;
startPos = nextPos + 2;
continue;
} else {
nextPos = sourceCode.length();
int commentStartPos = sourceCode.indexOf("/*", startPos);
int lineCommentStartPos = sourceCode.indexOf("//", startPos);
if (lineCommentStartPos != -1 && (commentStartPos == -1 || lineCommentStartPos < commentStartPos)) {
nextPos = lineCommentStartPos;
}
if (commentStartPos != -1 && (lineCommentStartPos == -1 || commentStartPos < lineCommentStartPos)) {
nextPos = commentStartPos;
inComment = true;
}
for (String keyword : keywords) {
int keywordPos = sourceCode.indexOf(keyword, startPos);
if (keywordPos != -1 && keywordPos < nextPos) {
int endPos = keywordPos + keyword.length();
if (endPos == sourceCode.length() || !Character.isJavaIdentifierPart(sourceCode.charAt(endPos))) {
keywordCountMap.put(keyword, keywordCountMap.getOrDefault(keyword, 0) + 1);
}
}
}
}
startPos = nextPos + 1;
}
for (Map.Entry<String, Integer> entry : keywordCountMap.entrySet()) {
System.out.printf("%d\t%s\n", entry.getValue(), entry.getKey());
}
}
}
```
该程序首先从标准输入读取输入的Java源码,然后使用一个字符串数组保存Java的关键字。接着,程序使用一个`Map`对象统计源码中出现的每个关键字的数量,并在统计时忽略了注释和字符串中出现的关键字。最后,程序按照关键字升序进行排序输出统计结果。
阅读全文
相关推荐
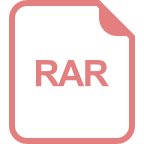
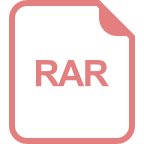














