使用python3.9,写一个Bert-BiLSTM-CRF模型的命名实体识别
时间: 2023-02-24 16:45:49 浏览: 371
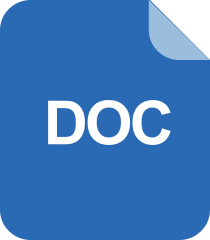
基于智能温度监测系统设计.doc
下面是一份使用 python 3.9 实现 Bert-BiLSTM-CRF 模型的命名实体识别的简单代码示例:
```python
import torch
import torch.nn as nn
from transformers import BertModel
class BertBiLSTMCRF(nn.Module):
def __init__(self, tag_to_ix, hidden_dim=768, num_layers=2, bidirectional=True, dropout=0.5):
super(BertBiLSTMCRF, self).__init__()
self.bert = BertModel.from_pretrained('bert-base-uncased')
self.lstm = nn.LSTM(hidden_dim, hidden_dim // 2, num_layers=num_layers, bidirectional=bidirectional, dropout=dropout)
self.fc = nn.Linear(hidden_dim, len(tag_to_ix))
self.crf = nn.CRF(len(tag_to_ix), batch_first=True)
self.hidden_dim = hidden_dim
self.tag_to_ix = tag_to_ix
self.tagset_size = len(tag_to_ix)
def forward(self, sent, tags=None):
with torch.no_grad():
bert_outputs = self.bert(sent)[0]
lstm_output, _ = self.lstm(bert_outputs)
feats = self.fc(lstm_output)
if tags is not None:
loss = -self.crf(feats, tags)
return loss
else:
scores = self.crf.forward_scores(feats)
return scores
def predict(self, sent):
scores = self.forward(sent)
_, predict_tags = scores.max(2)
return predict_tags
```
该代码示例中,我们使用了 BertModel 来构建 BERT 的预训练模型,并将其作为 BiLSTM 的输入,最后通过全连接层和 CRF 层实现命名实体识别。
请注意,这仅是一个简单的示例,你可能需要根据实际需求进行修改和优化。
阅读全文
相关推荐
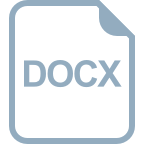
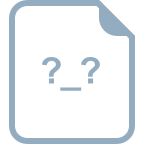
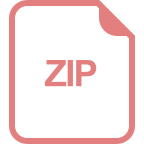
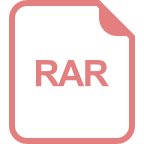
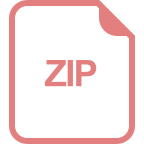
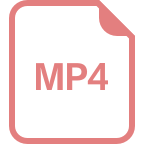
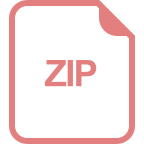
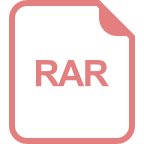
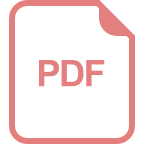