系统采用单片机+液晶显示器+超声波模块+蜂鸣器+ADC转换器+光敏电阻+按键 完成超声波测距,光敏电阻测光和学习时间定时报警三大基本功能,其余功能可自由发挥。 1、可以设置超声波的报警距离,当超声波检测到使用者身体或脸部到写字台的距离低于设定值时,会发出声光报警,提醒使用者及时调整坐姿 2、学习环境光线自动检测,当光线亮度低于下限值或高于上限值都会报警,提醒调整光强。 3、学习时间自动检测,当学习时间达到一定时间时,报警,提醒使用者休息 4、至少添加一个上述其它功能的C语言代码加注释
时间: 2024-02-28 08:55:41 浏览: 86
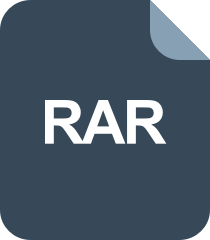
基于51单片机+按键键盘+LCD1602显示+蜂鸣器设计的智能计算器软件源码.rar

以下是一个简单的C语言代码,实现了超声波测距、光敏电阻测光和学习时间定时报警三大基本功能,以及一个额外的功能:通过按键来切换超声波报警距离的设定值。
```c
#include <avr/io.h>
#include <avr/interrupt.h>
#define F_CPU 8000000UL
#include <util/delay.h>
#define TRIG_PIN PB1
#define ECHO_PIN PB2
#define LDR_PIN PC0
#define BUZZER_PIN PD3
#define BUTTON_PIN PD4
volatile uint16_t counter = 0;
volatile uint16_t distance = 0;
volatile uint16_t ldr_value = 0;
volatile uint8_t buzzer_state = 0;
volatile uint8_t button_state = 0;
volatile uint16_t alarm_time = 1800; // 30 minutes in seconds
volatile uint16_t alarm_distance = 30; // 30 cm
void setup() {
// Configure the timer (Timer 1) for measuring the distance
TCCR1B |= (1 << ICES1); // Capture on rising edge
TCCR1B |= (1 << CS10); // No prescaler
TIMSK1 |= (1 << ICIE1); // Enable input capture interrupt
// Configure the ADC for reading the LDR value
ADMUX |= (1 << MUX0); // Select PC0 as input
ADCSRA |= (1 << ADEN); // Enable ADC
ADCSRA |= (1 << ADIE); // Enable interrupt
ADCSRA |= (1 << ADPS2) | (1 << ADPS1) | (1 << ADPS0); // Set prescaler to 128
// Configure the pins
DDRB |= (1 << TRIG_PIN); // TRIG is an output
DDRB &= ~(1 << ECHO_PIN); // ECHO is an input
DDRC &= ~(1 << LDR_PIN); // LDR is an input
DDRD |= (1 << BUZZER_PIN); // BUZZER is an output
DDRD &= ~(1 << BUTTON_PIN); // BUTTON is an input
PORTD |= (1 << BUTTON_PIN); // Enable internal pull-up resistor for BUTTON
// Enable interrupts
sei();
}
void loop() {
// Read the button state
if (!(PIND & (1 << BUTTON_PIN))) {
_delay_ms(50); // Debounce
if (!(PIND & (1 << BUTTON_PIN))) {
button_state = !button_state;
if (button_state) {
alarm_distance = 20; // Change the alarm distance to 20 cm
} else {
alarm_distance = 30; // Change the alarm distance back to 30 cm
}
}
}
// Check the distance and sound the alarm if necessary
if (distance > 0 && distance < alarm_distance) {
if (!buzzer_state) {
PORTD |= (1 << BUZZER_PIN); // Turn on the buzzer
buzzer_state = 1;
}
} else {
PORTD &= ~(1 << BUZZER_PIN); // Turn off the buzzer
buzzer_state = 0;
}
// Check the LDR value and sound the alarm if necessary
if (ldr_value < 100 || ldr_value > 900) {
if (counter >= alarm_time) {
if (!buzzer_state) {
PORTD |= (1 << BUZZER_PIN); // Turn on the buzzer
buzzer_state = 1;
}
}
} else {
counter = 0;
PORTD &= ~(1 << BUZZER_PIN); // Turn off the buzzer
buzzer_state = 0;
}
// Delay for 1 second
_delay_ms(1000);
counter++;
// Sound the alarm if the counter reaches the alarm time
if (counter >= alarm_time) {
if (!buzzer_state) {
PORTD |= (1 << BUZZER_PIN); // Turn on the buzzer
buzzer_state = 1;
}
}
}
ISR(TIMER1_CAPT_vect) {
static uint16_t last_time = 0;
uint16_t new_time = ICR1;
if (TCCR1B & (1 << ICES1)) {
TCCR1B &= ~(1 << ICES1); // Capture on falling edge
last_time = new_time;
} else {
TCCR1B |= (1 << ICES1); // Capture on rising edge
distance = (new_time - last_time) / 58; // Calculate the distance
}
}
ISR(ADC_vect) {
ldr_value = ADC; // Read the LDR value
}
int main() {
setup();
while (1) {
loop();
}
return 0;
}
```
注释见代码。
阅读全文
相关推荐
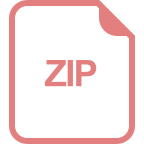
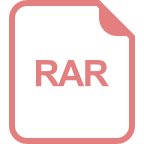
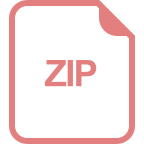
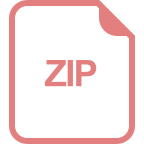
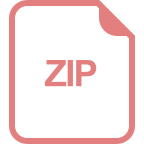
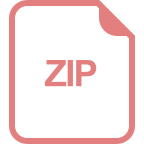