java从json数组中提取数据,从JSON数组中提取数据
时间: 2023-09-11 09:07:49 浏览: 67
可以使用Java中的JSON库,例如Jackson或Gson来从JSON数组中提取数据。以下是一个示例代码:
假设有以下JSON数组:
```
[
{
"name": "Alice",
"age": 25
},
{
"name": "Bob",
"age": 30
}
]
```
使用Jackson库:
```java
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
// jsonStr 是包含上述 JSON 数组的字符串
String jsonStr = "[{\"name\":\"Alice\",\"age\":25},{\"name\":\"Bob\",\"age\":30}]";
ObjectMapper objectMapper = new ObjectMapper();
JsonNode jsonNode = objectMapper.readTree(jsonStr);
// 遍历 JSON 数组,提取每个对象的数据
for (JsonNode node : jsonNode) {
String name = node.get("name").asText();
int age = node.get("age").asInt();
System.out.println("Name: " + name + ", Age: " + age);
}
```
使用Gson库:
```java
import com.google.gson.Gson;
import com.google.gson.JsonArray;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
// jsonStr 是包含上述 JSON 数组的字符串
String jsonStr = "[{\"name\":\"Alice\",\"age\":25},{\"name\":\"Bob\",\"age\":30}]";
Gson gson = new Gson();
JsonArray jsonArray = gson.fromJson(jsonStr, JsonArray.class);
// 遍历 JSON 数组,提取每个对象的数据
for (JsonElement element : jsonArray) {
JsonObject jsonObject = element.getAsJsonObject();
String name = jsonObject.get("name").getAsString();
int age = jsonObject.get("age").getAsInt();
System.out.println("Name: " + name + ", Age: " + age);
}
```
相关推荐
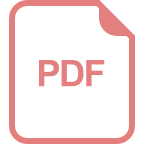
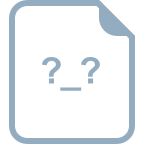
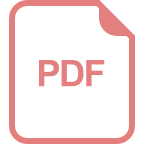
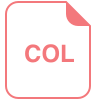
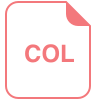
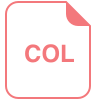
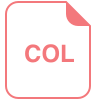
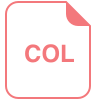









