在kotlin中使用springboot ,有一个controller方法: @PostMapping("/yz") fun yz(@RequestBody idVo: IdVo): IdVo { return idVo } 入参实体数据类是: data class IdVo( val id: String ) 我希望在属性id上加一个@DecryptId自定义注解,在进入controller之前,将IdVo的属性id进行解密操作。
时间: 2024-03-25 09:38:15 浏览: 19
首先,你需要定义一个`@DecryptId`注解,如下所示:
```kotlin
@Target(AnnotationTarget.FIELD)
@Retention(AnnotationRetention.RUNTIME)
annotation class DecryptId
```
然后,你需要定义一个拦截器来处理`@DecryptId`注解。该拦截器的作用是在进入controller之前,将`IdVo`的属性`id`进行解密操作。假设你使用的是AES对称加密算法,解密的密钥为`key`,解密的偏移量为`iv`,那么你的拦截器可能如下所示:
```kotlin
@Component
class DecryptIdInterceptor : HandlerInterceptor {
@Throws(Exception::class)
override fun preHandle(request: HttpServletRequest, response: HttpServletResponse, handler: Any): Boolean {
if (handler is HandlerMethod) {
val method = handler.method
val parameters = method.parameters
for (i in parameters.indices) {
val parameter = parameters[i]
if (parameter.isAnnotationPresent(RequestBody::class.java)) {
val arg = (handler.arguments[i] as HttpInputMessage).body.readBytes()
val objectMapper = ObjectMapper()
val idVo = objectMapper.readValue(arg, IdVo::class.java)
val fields = idVo.javaClass.declaredFields
for (field in fields) {
if (field.isAnnotationPresent(DecryptId::class.java)) {
field.isAccessible = true
val encryptedId = field.get(idVo) as String
val decryptedId = decrypt(encryptedId, key, iv)
field.set(idVo, decryptedId)
}
}
handler.arguments[i] = idVo
}
}
}
return true
}
private fun decrypt(encryptedText: String, key: String, iv: String): String {
val cipher = Cipher.getInstance("AES/CBC/PKCS5Padding")
val secretKeySpec = SecretKeySpec(key.toByteArray(charset("UTF-8")), "AES")
val ivParameterSpec = IvParameterSpec(iv.toByteArray(charset("UTF-8")))
cipher.init(Cipher.DECRYPT_MODE, secretKeySpec, ivParameterSpec)
val decryptedBytes = cipher.doFinal(Base64.getDecoder().decode(encryptedText))
return String(decryptedBytes, Charset.forName("UTF-8"))
}
}
```
最后,你需要在你的`@PostMapping`注解上添加一个`@RequestMapping`注解,将拦截器应用到该controller方法中,如下所示:
```kotlin
@PostMapping("/yz")
@RequestMapping(consumes = ["application/json"], produces = ["application/json"])
fun yz(@DecryptId @RequestBody idVo: IdVo): IdVo {
return idVo
}
```
现在,当你调用`/yz`接口时,`DecryptIdInterceptor`拦截器会自动将`IdVo`的属性`id`进行解密操作,然后进入controller方法中。
相关推荐
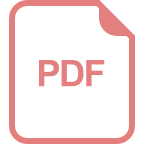
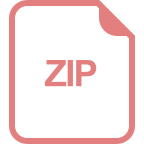
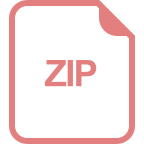















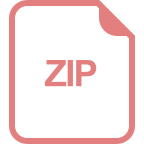