写一个充满科技感的钟表,并列出如何加入jsp页面中
时间: 2024-02-01 19:16:58 浏览: 21
1. 首先,我们可以使用HTML5和CSS3的一些新特性来创建一个充满科技感的钟表。
HTML代码:
```
<div class="clock">
<div class="hour-hand"></div>
<div class="minute-hand"></div>
<div class="second-hand"></div>
<div class="center-point"></div>
</div>
```
CSS代码:
```
.clock {
position: relative;
width: 200px;
height: 200px;
border-radius: 50%;
border: 2px solid #fff;
box-shadow: inset 0 0 10px rgba(0,0,0,0.1);
}
.hour-hand,
.minute-hand,
.second-hand {
position: absolute;
background: #fff;
border-radius: 2px;
transform-origin: bottom center;
}
.hour-hand {
width: 4px;
height: 50px;
top: 25px;
left: 98px;
}
.minute-hand {
width: 3px;
height: 70px;
top: 15px;
left: 99px;
}
.second-hand {
width: 2px;
height: 80px;
top: 10px;
left: 100px;
}
.center-point {
position: absolute;
width: 6px;
height: 6px;
border-radius: 50%;
background: #fff;
top: 97px;
left: 97px;
}
/* 动画效果 */
@keyframes rotate {
from {
transform: rotate(0deg);
}
to {
transform: rotate(360deg);
}
}
.second-hand {
animation: rotate 60s linear infinite;
}
.minute-hand {
animation: rotate 3600s linear infinite;
}
.hour-hand {
animation: rotate 43200s linear infinite;
}
```
这个代码块将会在浏览器中展示出一个基本的钟表,包括时针、分针、秒针和中心点。
2. 将这个代码块保存为一个单独的 HTML 文件,例如 clock.html。
3. 在 JSP 页面中添加以下代码来引用 clock.html 文件:
```
<%@include file="clock.html" %>
```
这将在 JSP 页面中嵌入 clock.html 文件的内容,包括我们刚刚创建的钟表。
4. 如果你想在页面中调整钟表的大小或位置,可以使用 CSS 来修改 .clock 类的属性。例如:
```
.clock {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
width: 300px;
height: 300px;
}
```
这将把钟表放置在页面的中心,同时将它的大小设置为 300x300 像素。
5. 最后,你可以使用 JavaScript 来动态地控制钟表的行为。例如,你可以使用 setInterval() 函数来每秒更新秒针的位置:
```
setInterval(function() {
var date = new Date();
var seconds = date.getSeconds();
var degrees = seconds * 6;
document.querySelector(".second-hand").style.transform = "rotate(" + degrees + "deg)";
}, 1000);
```
这将每秒钟更新一次秒针的位置,使它沿着钟表的轨道旋转。
相关推荐
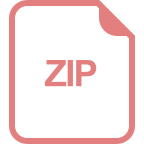














