如何通过selenium自动化控制鼠标下滑
时间: 2023-09-07 14:13:16 浏览: 102
你可以使用Selenium的ActionChains类来模拟鼠标操作,其中包括滚动鼠标的下拉操作。
以下是一个示例代码,可以在浏览器中模拟向下滚动鼠标的操作:
```
from selenium.webdriver.common.action_chains import ActionChains
# 假设你已经启动了一个浏览器并且加载了一个网页
driver = webdriver.Chrome()
# 定位到页面底部元素
bottom_element = driver.find_element_by_xpath("//footer")
# 创建ActionChains对象
actions = ActionChains(driver)
# 将鼠标移动到底部元素
actions.move_to_element(bottom_element)
# 执行滚动操作
actions.click_and_hold().move_by_offset(0, 100).release().perform()
```
在上述代码中,我们首先定位到页面底部的元素(例如 footer 标签),然后使用 ActionChains 对象来模拟鼠标操作。在这个示例中,我们使用 `click_and_hold()` 方法来模拟按住鼠标左键,然后使用 `move_by_offset()` 方法来向下移动鼠标,最后使用 `release()` 方法来释放鼠标左键。`perform()` 方法用于执行操作。
你可以根据页面的具体情况和滑动需求进行调整。
相关问题
如何通过selenium自动化控制页面下滑
通过Selenium可以模拟用户的浏览行为,从而实现页面下滑的自动化控制。
可以使用以下代码实现页面的下滑:
```python
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
driver = webdriver.Chrome()
driver.get("https://www.example.com")
# 定位到页面底部
html = driver.find_element_by_tag_name('html')
html.send_keys(Keys.END)
```
这段代码使用Chrome浏览器打开了一个网页,然后通过定位到`<html>`标签,在页面中模拟按下End键,从而实现了页面的下滑。
也可以通过循环多次按下End键,实现下滑多次的效果:
```python
for i in range(5):
html.send_keys(Keys.END)
time.sleep(1)
```
这段代码将页面下滑了5次,每次下滑之后等待1秒钟,从而模拟用户的操作。
selenium自动化测试鼠标操作
Selenium可以模拟用户对网页进行鼠标操作,如单击、双击、右击、拖拽等。以下是几个常用的鼠标操作示例:
1. 单击:
```python
from selenium.webdriver.common.action_chains import ActionChains
# 找到需要单击的元素
element = driver.find_element_by_xpath("//button[@id='xxx']")
# 对元素执行单击操作
ActionChains(driver).click(element).perform()
```
2. 双击:
```python
from selenium.webdriver.common.action_chains import ActionChains
# 找到需要双击的元素
element = driver.find_element_by_xpath("//button[@id='xxx']")
# 对元素执行双击操作
ActionChains(driver).double_click(element).perform()
```
3. 右击:
```python
from selenium.webdriver.common.action_chains import ActionChains
# 找到需要右击的元素
element = driver.find_element_by_xpath("//button[@id='xxx']")
# 对元素执行右击操作
ActionChains(driver).context_click(element).perform()
```
4. 拖拽:
```python
from selenium.webdriver.common.action_chains import ActionChains
# 找到需要拖拽的元素
source_element = driver.find_element_by_xpath("//div[@id='source']")
target_element = driver.find_element_by_xpath("//div[@id='target']")
# 对元素执行拖拽操作
ActionChains(driver).drag_and_drop(source_element, target_element).perform()
```
以上是常用的鼠标操作示例,你可以根据需要进行组合和扩展。
相关推荐
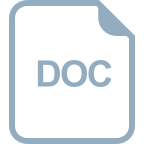
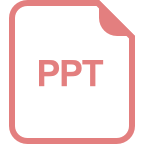
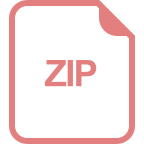
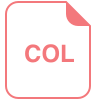
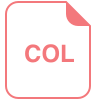
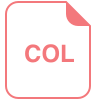
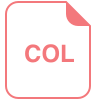
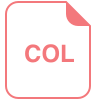







