1. 编写一个函数Print,能够打印多个学生的各门成绩,在主函数中定义一个可以存放有5个学生数据的数组。每个学生的数据包括num(学号),name(姓名),score[3](3门课的成绩)。在主函数输入这些数据,用Print函数输出这些数据。(结构体类型编写) 2. 在上一题的基础上,编写一个函数SortStud对5个学生按每个学生的3门成绩总分(由高到低)进行排序,并用new申请存放5个学生数据的存储空间。
时间: 2024-04-06 17:32:54 浏览: 93
好的,我会根据你的要求进行回答。以下是你的问题的答案:
1. 首先,我们需要定义一个结构体类型来储存每个学生的信息:
```c++
struct Student {
int num; // 学号
string name; // 姓名
float score[3]; // 3门课的成绩
};
```
然后,在主函数中定义一个包含5个学生数据的数组,并输入这些数据:
```c++
int main() {
Student students[5];
for (int i = 0; i < 5; i++) {
cout << "请输入第" << i+1 << "个学生的信息:" << endl;
cout << "学号:";
cin >> students[i].num;
cout << "姓名:";
cin >> students[i].name;
cout << "3门课的成绩:";
for (int j = 0; j < 3; j++) {
cin >> students[i].score[j];
}
}
// 调用Print函数输出数据
Print(students, 5);
return 0;
}
```
最后,定义Print函数来输出数据:
```c++
void Print(Student students[], int n) {
cout << "学号\t姓名\t课程1\t课程2\t课程3" << endl;
for (int i = 0; i < n; i++) {
cout << students[i].num << "\t" << students[i].name << "\t";
for (int j = 0; j < 3; j++) {
cout << students[i].score[j] << "\t";
}
cout << endl;
}
}
```
2. 在上一题的基础上,我们需要定义一个新的函数SortStud来对学生按照总分进行排序,并用new申请一个新的存储空间:
```c++
Student* SortStud(Student students[], int n) {
// 用new申请一个新的存储空间
Student* newStudents = new Student[n];
// 复制原数组
for (int i = 0; i < n; i++) {
newStudents[i] = students[i];
}
// 冒泡排序
for (int i = 0; i < n-1; i++) {
for (int j = 0; j < n-i-1; j++) {
float sum1 = newStudents[j].score[0] + newStudents[j].score[1] + newStudents[j].score[2];
float sum2 = newStudents[j+1].score[0] + newStudents[j+1].score[1] + newStudents[j+1].score[2];
if (sum1 < sum2) {
swap(newStudents[j], newStudents[j+1]);
}
}
}
return newStudents;
}
```
然后,在主函数中调用SortStud函数并输出排序后的结果:
```c++
int main() {
Student students[5];
// 输入数据
// 调用Print函数输出原数据
Print(students, 5);
// 调用SortStud函数进行排序
Student* newStudents = SortStud(students, 5);
// 调用Print函数输出排序后的结果
Print(newStudents, 5);
// 释放新数组的内存
delete[] newStudents;
return 0;
}
```
阅读全文
相关推荐


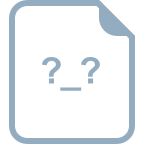









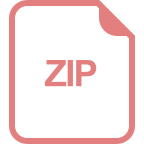
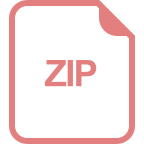
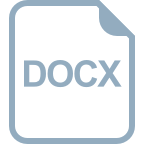
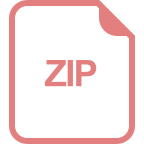
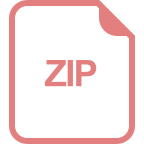