Java程序部署在服务器上,如何用java让服务器从另一台服务器上复制一个文件夹
时间: 2023-05-13 18:04:29 浏览: 87
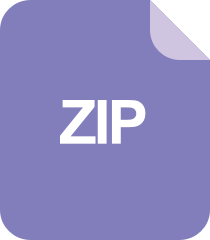
java实现两台服务器间文件复制的方法
您可以使用Java中的File类和IO流来实现从另一台服务器上复制一个文件夹。具体步骤如下:
1. 创建一个File对象,指定要复制的文件夹路径。
2. 使用File类的listFiles()方法获取该文件夹下的所有文件和子文件夹。
3. 遍历所有文件和子文件夹,如果是文件夹则递归调用复制方法,如果是文件则使用IO流将文件复制到目标服务器上。
4. 复制完成后关闭IO流。
以下是示例代码:
```
import java.io.*;
public class CopyFolder {
public static void main(String[] args) {
String sourceFolder = "/path/to/source/folder";
String targetFolder = "/path/to/target/folder";
File source = new File(sourceFolder);
File target = new File(targetFolder);
copyFolder(source, target);
}
public static void copyFolder(File source, File target) {
if (source.isDirectory()) {
if (!target.exists()) {
target.mkdir();
}
String[] files = source.list();
for (String file : files) {
File srcFile = new File(source, file);
File tgtFile = new File(target, file);
copyFolder(srcFile, tgtFile);
}
} else {
try (InputStream in = new FileInputStream(source);
OutputStream out = new FileOutputStream(target)) {
byte[] buffer = new byte[1024];
int length;
while ((length = in.read(buffer)) > 0) {
out.write(buffer, 0, length);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
```
阅读全文
相关推荐

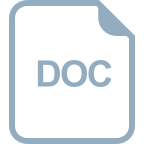
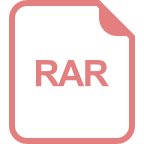
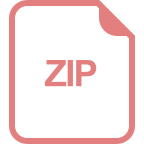
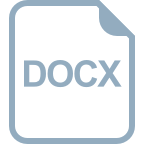
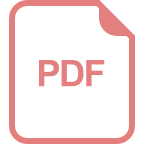
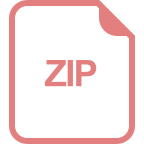
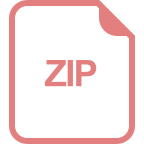
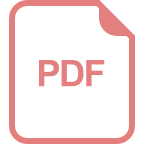
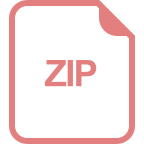
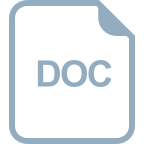
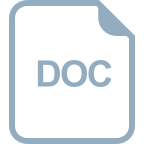
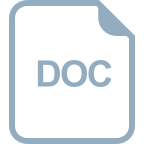
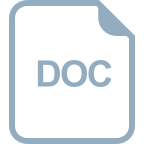
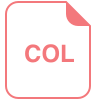