SpringMVC框架的使用实现使用拦截器强制未登录用户进行登录和登录用户可进行文件的上传和下载的代码
时间: 2024-05-14 15:17:59 浏览: 18
以下是使用拦截器实现强制登录和文件上传下载的示例代码:
1. 编写拦截器
```java
public class LoginInterceptor implements HandlerInterceptor {
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception {
HttpSession session = request.getSession();
// 判断用户是否登录
if (session.getAttribute("user") == null) {
// 未登录,重定向到登录页面
response.sendRedirect(request.getContextPath() + "/login");
return false;
}
return true;
}
}
```
2. 配置拦截器
```xml
<mvc:interceptors>
<mvc:interceptor>
<mvc:mapping path="/**"/>
<mvc:exclude-mapping path="/login"/> <!-- 排除登录页面的拦截 -->
<bean class="com.example.interceptor.LoginInterceptor"/>
</mvc:interceptor>
</mvc:interceptors>
```
3. 编写文件上传和下载的控制器
```java
@Controller
public class FileController {
@RequestMapping("/upload")
public String upload() {
return "upload";
}
@RequestMapping(value = "/upload", method = RequestMethod.POST)
public String upload(@RequestParam("file") MultipartFile file, HttpServletRequest request) throws IOException {
if (!file.isEmpty()) {
// 获取上传文件的原始文件名
String fileName = file.getOriginalFilename();
// 获取上传文件的保存路径
String filePath = request.getSession().getServletContext().getRealPath("/upload/");
// 创建保存文件的目录
File dir = new File(filePath);
if (!dir.exists()) {
dir.mkdirs();
}
// 保存上传文件
file.transferTo(new File(filePath + fileName));
return "redirect:/upload";
}
return "redirect:/error";
}
@RequestMapping("/download/{fileName:.+}")
public void download(@PathVariable String fileName, HttpServletRequest request, HttpServletResponse response) throws IOException {
// 获取下载文件的保存路径
String filePath = request.getSession().getServletContext().getRealPath("/upload/");
// 根据文件名创建一个文件对象
File file = new File(filePath + fileName);
// 设置响应头信息
response.setContentType("application/octet-stream");
response.setHeader("Content-Disposition", "attachment;filename=" + new String(fileName.getBytes("UTF-8"), "ISO-8859-1"));
// 将文件读取到输入流中
InputStream in = new FileInputStream(file);
// 将输入流中的数据写入到输出流中
OutputStream out = response.getOutputStream();
byte[] buffer = new byte[1024];
int len;
while ((len = in.read(buffer)) > 0) {
out.write(buffer, 0, len);
}
// 关闭输入输出流
in.close();
out.close();
}
}
```
在上述控制器中,`/upload`请求用于展示上传文件的页面,`/upload` POST请求用于处理文件上传,`/download/{fileName:.+}`请求用于处理文件下载,其中`{fileName:.+}`表示可以匹配包含点号的文件名。
4. 编写上传文件的页面
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>文件上传</title>
</head>
<body>
<form method="post" action="/upload" enctype="multipart/form-data">
<input type="file" name="file"/>
<input type="submit" value="上传"/>
</form>
</body>
</html>
```
5. 配置文件上传保存路径
在`applicationContext.xml`中配置文件上传保存路径:
```xml
<bean id="multipartResolver" class="org.springframework.web.multipart.commons.CommonsMultipartResolver">
<property name="maxUploadSize" value="5242880"/>
<property name="defaultEncoding" value="UTF-8"/>
<property name="resolveLazily" value="true"/>
</bean>
<mvc:resources mapping="/upload/**" location="/upload/"/>
<bean id="multipartConfigElement" class="javax.servlet.annotation.MultipartConfigElement">
<property name="location" value="/upload/"/>
</bean>
```
以上就是使用拦截器实现强制登录和文件上传下载的示例代码。请注意,这仅仅是一个示例,实际项目中需要根据具体需求进行修改和完善。
相关推荐
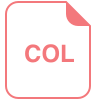
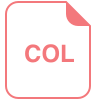
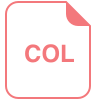
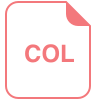
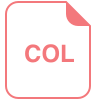









