windows下用C++实现SCSI READ一次命令读取512K数据代码
时间: 2024-03-05 16:54:42 浏览: 187
以下是一个简单的 C++ 代码示例,用于在 Windows 环境下使用 SCSI READ 命令读取 512K 数据:
```c++
#include <windows.h>
#include <winioctl.h>
#define SECTOR_SIZE 512
#define BUFFER_SIZE (SECTOR_SIZE * 1024)
int main()
{
HANDLE hDevice = CreateFile("\\\\.\\PhysicalDrive0", GENERIC_READ, FILE_SHARE_READ | FILE_SHARE_WRITE,
NULL, OPEN_EXISTING, 0, NULL);
if (hDevice == INVALID_HANDLE_VALUE)
{
printf("Failed to open device, error code: %d\n", GetLastError());
return 1;
}
BYTE buffer[BUFFER_SIZE] = { 0 };
DWORD bytesReturned = 0;
STORAGE_PROPERTY_QUERY storagePropertyQuery = { 0 };
STORAGE_DESCRIPTOR_HEADER storageDescriptorHeader = { 0 };
storagePropertyQuery.PropertyId = StorageAdapterProperty;
storagePropertyQuery.QueryType = PropertyStandardQuery;
if (!DeviceIoControl(hDevice, IOCTL_STORAGE_QUERY_PROPERTY,
&storagePropertyQuery, sizeof(storagePropertyQuery), &storageDescriptorHeader,
sizeof(storageDescriptorHeader), &bytesReturned, NULL))
{
printf("Failed to retrieve adapter property, error code: %d\n", GetLastError());
CloseHandle(hDevice);
return 1;
}
STORAGE_READ_CAPACITY readCapacity = { 0 };
if (!DeviceIoControl(hDevice, IOCTL_STORAGE_READ_CAPACITY, NULL, 0, &readCapacity,
sizeof(readCapacity), &bytesReturned, NULL))
{
printf("Failed to retrieve read capacity, error code: %d\n", GetLastError());
CloseHandle(hDevice);
return 1;
}
DWORD sectorCount = readCapacity.DiskLength.QuadPart / SECTOR_SIZE;
DWORD startingSector = 0;
if (!DeviceIoControl(hDevice, IOCTL_STORAGE_CHECK_VERIFY, NULL, 0, NULL, 0,
&bytesReturned, NULL))
{
printf("Failed to verify device, error code: %d\n", GetLastError());
CloseHandle(hDevice);
return 1;
}
SCSI_PASS_THROUGH_DIRECT_WITH_BUFFER sptdwb = { 0 };
sptdwb.sptd.Length = sizeof(SCSI_PASS_THROUGH_DIRECT);
sptdwb.sptd.PathId = 0;
sptdwb.sptd.TargetId = 0;
sptdwb.sptd.Lun = 0;
sptdwb.sptd.TimeOutValue = 2;
sptdwb.sptd.DataIn = SCSI_IOCTL_DATA_IN;
sptdwb.sptd.DataTransferLength = BUFFER_SIZE;
sptdwb.sptd.DataBuffer = buffer;
sptdwb.sptd.SenseInfoLength = SPT_SENSE_LENGTH;
sptdwb.sptd.CdbLength = 10;
sptdwb.sptd.SenseInfoOffset = offsetof(SCSI_PASS_THROUGH_DIRECT_WITH_BUFFER, SenseBuf);
sptdwb.sptd.Cdb[0] = 0x28; // SCSI READ command
sptdwb.sptd.Cdb[1] = 0;
sptdwb.sptd.Cdb[2] = (startingSector >> 24) & 0xFF;
sptdwb.sptd.Cdb[3] = (startingSector >> 16) & 0xFF;
sptdwb.sptd.Cdb[4] = (startingSector >> 8) & 0xFF;
sptdwb.sptd.Cdb[5] = startingSector & 0xFF;
sptdwb.sptd.Cdb[6] = 0;
sptdwb.sptd.Cdb[7] = (BUFFER_SIZE / SECTOR_SIZE) & 0xFF;
sptdwb.sptd.Cdb[8] = 0;
sptdwb.sptd.Cdb[9] = 0;
if (!DeviceIoControl(hDevice, IOCTL_SCSI_PASS_THROUGH_DIRECT, &sptdwb,
sizeof(SCSI_PASS_THROUGH_DIRECT_WITH_BUFFER), &sptdwb,
sizeof(SCSI_PASS_THROUGH_DIRECT_WITH_BUFFER), &bytesReturned, NULL))
{
printf("Failed to execute SCSI READ command, error code: %d\n", GetLastError());
CloseHandle(hDevice);
return 1;
}
CloseHandle(hDevice);
return 0;
}
```
请注意,这只是一个简单的示例代码,可能需要根据实际情况进行更改。同时,使用 SCSI 命令需要具有足够的权限,并且必须小心谨慎,以免意外损坏设备。
阅读全文
相关推荐
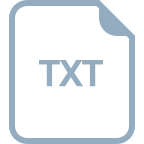
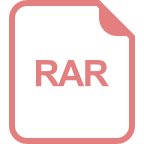
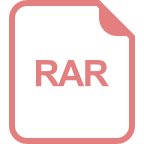
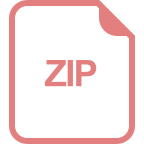
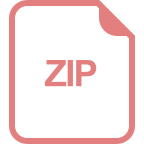
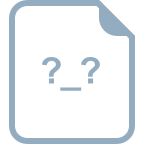
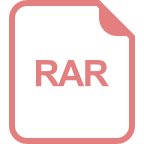
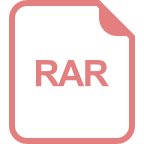
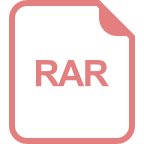
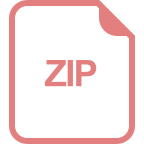
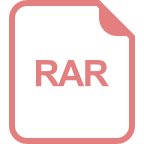
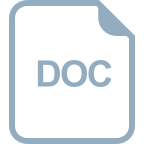
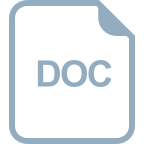
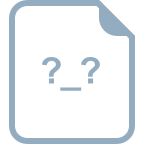