请用javafx设计一个财务收支业务的程序,构建存钱、取钱两个线程。 存钱线程启动后,每隔1秒钟,随机往账户中存入[0,20]块钱。 每次存钱程序输出:in+空格+存入金额+空格+账户余额 当金额超过100时,唤醒取钱线程, 取钱线程被唤醒后,随机在账户中提取[50-100]块钱。 每次取钱程序输出:out+空格+支取金额+空格+账户余额
时间: 2023-12-23 19:03:29 浏览: 93
以下是一个使用 JavaFX 实现的财务收支业务程序:
```java
import java.util.Random;
import javafx.application.Application;
import javafx.application.Platform;
import javafx.concurrent.Task;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class FinancialTransaction extends Application {
private static final Random RANDOM = new Random();
private static int balance = 0;
@Override
public void start(Stage stage) {
Label balanceLabel = new Label("Balance: " + balance);
StackPane root = new StackPane(balanceLabel);
Scene scene = new Scene(root, 200, 100);
stage.setScene(scene);
stage.show();
Thread depositThread = new Thread(new Task<Void>() {
@Override
protected Void call() throws Exception {
while (true) {
int amount = RANDOM.nextInt(21);
synchronized (this) {
balance += amount;
Platform.runLater(() -> balanceLabel.setText("in " + amount + " " + balance));
if (balance > 100) {
notify();
}
}
Thread.sleep(1000);
}
}
});
Thread withdrawThread = new Thread(new Task<Void>() {
@Override
protected Void call() throws Exception {
while (true) {
synchronized (depositThread) {
while (balance <= 100) {
depositThread.wait();
}
int amount = RANDOM.nextInt(51) + 50;
synchronized (this) {
balance -= amount;
Platform.runLater(() -> balanceLabel.setText("out " + amount + " " + balance));
}
}
Thread.sleep(1000);
}
}
});
depositThread.start();
withdrawThread.start();
}
public static void main(String[] args) {
launch();
}
}
```
该程序使用 JavaFX 构建 UI,显示账户余额。存钱线程和取钱线程分别实现了 Runnable 接口,使用 synchronized 和 wait/notify 保证线程安全。存钱线程每隔1秒钟随机往账户中存入一定金额,当账户余额超过100时,唤醒取钱线程。取钱线程被唤醒后,随机在账户中提取一定金额。每次存钱和取钱操作后,程序会更新 UI 上的账户余额信息。
阅读全文
相关推荐
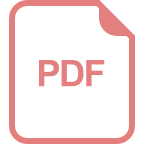
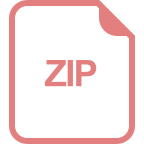
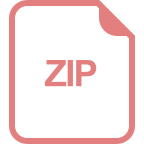



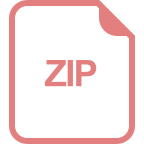
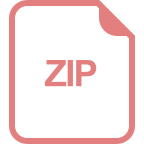
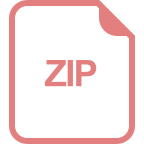
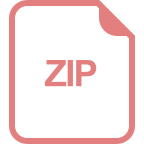
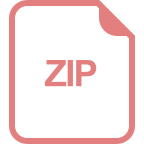
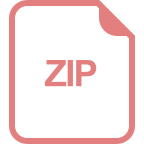
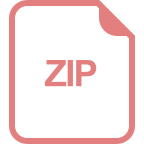
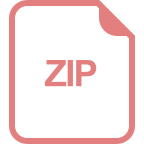
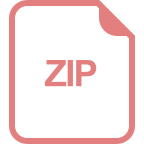