写一段stm32 comp out io实现比较器的例子代码
时间: 2023-03-04 17:33:50 浏览: 174
以下是使用STM32的COMP(比较器)模块和GPIO(通用输入/输出)模块实现比较器的示例代码。在本例中,将使用PA1引脚作为比较器的输入,并将PB0引脚作为输出,以指示比较器输出的状态。
```
#include "stm32f4xx.h"
int main(void)
{
// 初始化时钟
RCC_AHB1PeriphClockCmd(RCC_AHB1Periph_GPIOA | RCC_AHB1Periph_GPIOB, ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_SYSCFG, ENABLE);
// 配置PA1引脚为比较器输入
GPIO_InitTypeDef GPIO_InitStruct;
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_1;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AN;
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_Init(GPIOA, &GPIO_InitStruct);
// 配置PB0引脚为输出
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_0;
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_OUT;
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStruct.GPIO_OType = GPIO_OType_PP;
GPIO_InitStruct.GPIO_PuPd = GPIO_PuPd_NOPULL;
GPIO_Init(GPIOB, &GPIO_InitStruct);
// 配置比较器
COMP_InitTypeDef COMP_InitStruct;
COMP_StructInit(&COMP_InitStruct);
COMP_InitStruct.COMP_InvertingInput = COMP_InvertingInput_VREFINT;
COMP_InitStruct.COMP_NonInvertingInput = COMP_NonInvertingInput_IO1;
COMP_InitStruct.COMP_Output = COMP_Output_TIM1BKIN2;
COMP_InitStruct.COMP_OutputPol = COMP_OutputPol_NonInverted;
COMP_InitStruct.COMP_Hysteresis = COMP_Hysteresis_No;
COMP_Init(COMP_Selection_COMP1, &COMP_InitStruct);
// 启用比较器中断
COMP_ITConfig(COMP_Selection_COMP1, ENABLE);
NVIC_EnableIRQ(COMP_IRQn);
while (1)
{
// 此处可以添加其他代码或等待中断
}
}
// 比较器中断处理函数
void COMP_IRQHandler(void)
{
if (COMP_GetOutputLevel(COMP_Selection_COMP1) == COMP_OutputLevel_High)
{
// 如果比较器输出为高,则将PB0引脚设置为高电平
GPIO_SetBits(GPIOB, GPIO_Pin_0);
}
else
{
// 如果比较器输出为低,则将PB0引脚设置为低电平
GPIO_ResetBits(GPIOB, GPIO_Pin_0);
}
// 清除比较器中断标志
COMP_ClearITPendingBit(COMP_Selection_COMP1);
}
```
该代码使用PA1引脚作为比较器的输入,将其连接到外部电路中。在配置完比较器和GPIO模块后,启用了比较器中断,并实现了一个中断处理函数。当比较器输出电平变化时,中断处理函数将相应地更改PB0引脚的状态。
阅读全文
相关推荐
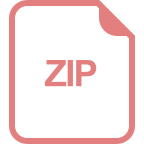
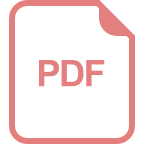
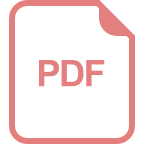
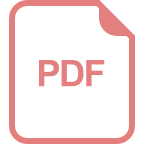
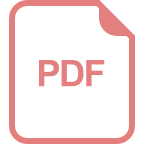
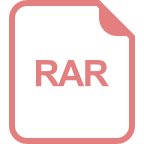
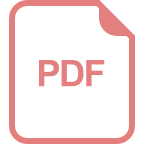
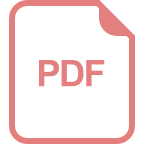
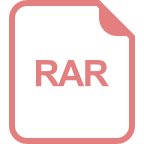
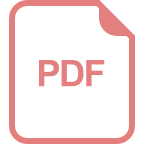
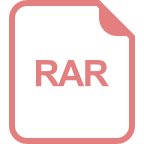
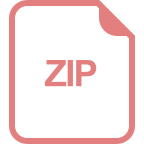
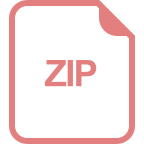
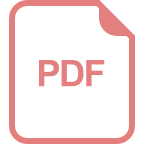
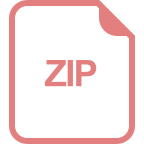