字符串长度c++怎么表示
时间: 2023-08-31 15:09:52 浏览: 96
在 C++ 中,可以使用 `std::string` 类型来表示字符串,该类型提供了许多便捷的操作方法,例如获取字符串长度可以使用 `length()` 或 `size()` 方法。例如:
```cpp
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
std::cout << str.length() << std::endl; // 输出:13
std::cout << str.size() << std::endl; // 输出:13
return 0;
}
```
除了使用 `std::string` 类型外,也可以使用 C 风格的字符串,即以空字符 '\0' 结尾的字符数组。通过调用 C++ 标准库中的 `strlen()` 函数可以获取该字符数组的长度。例如:
```cpp
#include <iostream>
#include <cstring>
int main() {
char str[] = "Hello, world!";
std::cout << strlen(str) << std::endl; // 输出:13
return 0;
}
```
阅读全文
相关推荐
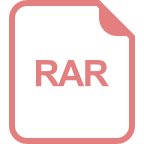
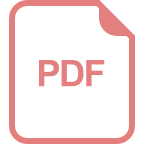
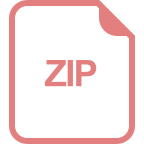
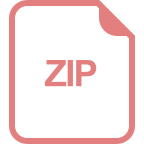
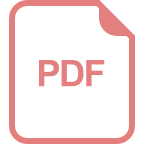
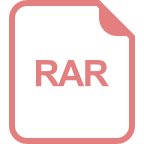
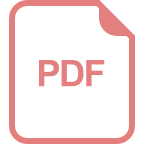
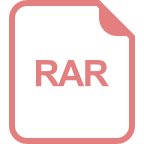
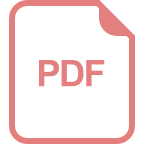
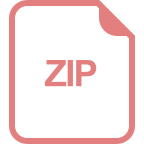
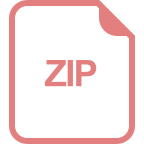
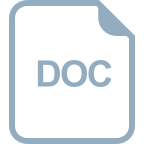
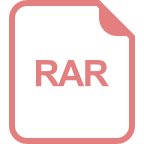
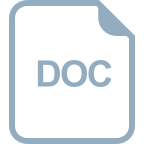
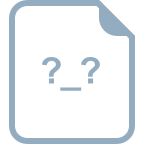
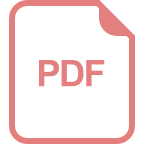