net.sf.json.JSONArray中的对象是Object,我在遍历net.sf.json.JSONArray的时候,怎样将里面的Object转成net.sf.json.JSONObject
时间: 2023-11-11 15:04:14 浏览: 135
你可以使用 `net.sf.json.JSONObject` 的 `fromObject()` 方法将 `Object` 对象转换为 `JSONObject` 对象。例如:
```
JSONArray jsonArray = new JSONArray();
JSONObject jsonObject1 = new JSONObject();
jsonObject1.put("name", "John");
jsonObject1.put("age", 30);
jsonArray.add(jsonObject1);
JSONObject jsonObject2 = new JSONObject();
jsonObject2.put("name", "Mary");
jsonObject2.put("age", 25);
jsonArray.add(jsonObject2);
for (Object object : jsonArray) {
JSONObject jsonObject = JSONObject.fromObject(object);
System.out.println(jsonObject.toString());
}
```
输出结果为:
```
{"name":"John","age":30}
{"name":"Mary","age":25}
```
在遍历 `JSONArray` 时,使用 `fromObject()` 方法将每个 `Object` 元素转换为 `JSONObject` 对象,然后进行处理。
相关问题
import net.sf.json.JSONArray; import net.sf.json.JSONObject;这两个导入怎么使用
`import net.sf.json.JSONArray;` 和 `import net.sf.json.JSONObject;` 这两个导入语句是用来使用 Apache Commons JSON 库中的两个重要类:JSONArray 和 JSONObject。在 Java 中,JSON (JavaScript Object Notation) 是一种轻量级的数据交换格式,常用于 Web 开发中的前后端数据交互。
1. JSONArray:这是一个用于存储有序 JSON 数组的对象,你可以创建它并添加元素(如字符串、数字等),然后通过索引来访问和操作数组中的项。例如:
```java
JSONArray jsonArray = new JSONArray();
jsonArray.add("item1");
jsonArray.add(10);
System.out.println(jsonArray.get(0)); // 输出 "item1"
```
2. JSONObject:类似于数组,但用于存储键值对形式的 JSON 对象。可以设置键值对,获取键对应的值,以及遍历对象的属性。示例:
```java
JSONObject jsonObject = new JSONObject();
jsonObject.put("name", "John");
jsonObject.put("age", 30);
String name = jsonObject.getString("name"); // 获取 "name" 的值
System.out.println(name); // 输出 "John"
```
要使用这两个类,确保已包含 Commons JSON 库到项目中,并在需要的地方导入它们。注意,Apache Commons JSON 已经不再维护,现在推荐使用更现代的 JSON 库,如 Jackson 或 Gson。
使用net.sf.jsonAPI
如果你想使用`net.sf.json`库来将JSON字符串转换为List,你可以按照以下步骤进行操作:
1. 首先,确保你已经将`net.sf.json`库添加到你的项目依赖中。你可以在 Maven 或者 Gradle 的配置文件中添加如下依赖:
```xml
<dependency>
<groupId>net.sf.json-lib</groupId>
<artifactId>json-lib</artifactId>
<version>2.4</version>
<classifier>jdk15</classifier>
</dependency>
```
2. 接下来,使用`JSONArray`类将JSON字符串转换为JSONArray对象,然后通过遍历JSONArray对象将其转换为List。以下是一个示例代码:
```java
import net.sf.json.JSONArray;
import net.sf.json.JSONObject;
import java.util.ArrayList;
import java.util.List;
public class JsonToListExample {
public static void main(String[] args) {
String jsonStr = "[{\"name\":\"John\",\"age\":30},{\"name\":\"Jane\",\"age\":25}]";
JSONArray jsonArray = JSONArray.fromObject(jsonStr);
List<Person> personList = new ArrayList<>();
for (Object obj : jsonArray) {
JSONObject jsonObject = (JSONObject) obj;
Person person = (Person) JSONObject.toBean(jsonObject, Person.class);
personList.add(person);
}
for (Person person : personList) {
System.out.println("Name: " + person.getName());
System.out.println("Age: " + person.getAge());
}
}
static class Person {
private String name;
private int age;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
}
```
在上面的示例中,我们首先使用`JSONArray.fromObject()`方法将JSON字符串转换为JSONArray对象。然后,通过遍历JSONArray对象,我们将每个JSONObject转换为Person对象,并将其添加到List中。
请确保在使用`net.sf.json`库之前,将其添加到你的项目依赖中。
阅读全文
相关推荐
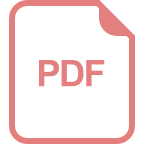
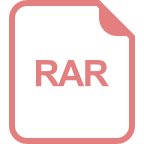
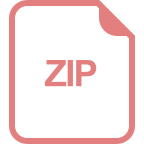
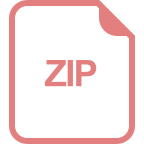
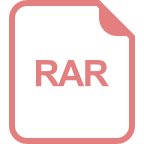
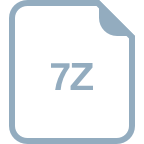
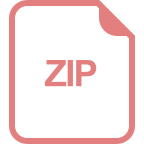
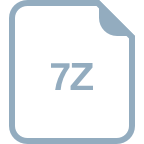
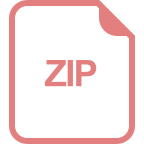
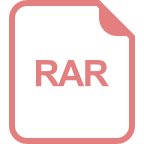
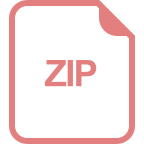
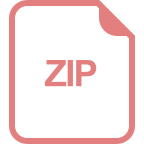

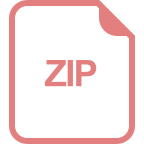
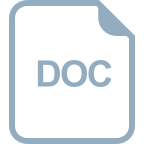