esp32 OTA micropython
时间: 2023-05-17 08:07:14 浏览: 340
可以使用 MicroPython 的 OTA(Over-The-Air)功能来更新 ESP32 的固件。以下是一个简单的示例代码:
```python
import network
import time
import urequests
import uos
import machine
import binascii
import ubinascii
import uhashlib
# WiFi 配置
ssid = 'your_wifi_ssid'
password = 'your_wifi_password'
# 固件更新地址
update_url = 'http://your_firmware_update_url'
# 获取 ESP32 的唯一标识符
def get_unique_id():
mac = ubinascii.hexlify(network.WLAN().config('mac'), ':').decode()
return uhashlib.sha256(mac.encode()).digest()
# 获取当前固件版本
def get_firmware_version():
with open('version.txt', 'r') as f:
version = f.read().strip()
return version
# 下载新固件
def download_firmware():
print('Downloading firmware...')
response = urequests.get(update_url)
with open('firmware.bin', 'wb') as f:
f.write(response.content)
response.close()
# 更新固件
def update_firmware():
print('Updating firmware...')
with open('firmware.bin', 'rb') as f:
firmware = f.read()
with open('firmware.bin', 'wb') as f:
f.write(b'')
with open('firmware.bin', 'wb') as f:
f.write(firmware)
machine.reset()
# 连接 WiFi
def connect_wifi():
print('Connecting to WiFi...')
sta_if = network.WLAN(network.STA_IF)
sta_if.active(True)
sta_if.connect(ssid, password)
while not sta_if.isconnected():
time.sleep(1)
print('WiFi connected:', sta_if.ifconfig())
# 主程序
def main():
connect_wifi()
unique_id = binascii.hexlify(get_unique_id()).decode()
print('ESP32 unique ID:', unique_id)
current_version = get_firmware_version()
print('Current firmware version:', current_version)
download_firmware()
new_version = get_firmware_version()
print('New firmware version:', new_version)
if new_version > current_version:
update_firmware()
else:
print('No firmware update available.')
if __name__ == '__main__':
main()
```
这个示例代码使用 MicroPython 的网络库和文件操作库来实现 OTA 功能。它首先连接到 WiFi,然后获取 ESP32 的唯一标识符和当前固件版本。接着它从指定的固件更新地址下载新固件,并检查新固件的版本是否比当前固件版本高。如果是,它将新固件写入 ESP32 的闪存,并重启 ESP32 来启动新固件。否则,它将不执行任何操作。
请注意,这个示例代码仅供参考,实际应用中可能需要根据具体情况进行修改和优化。
相关推荐
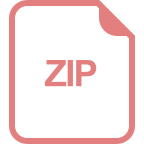
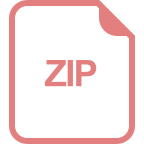









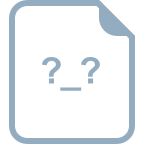
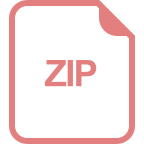
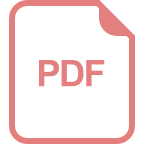