使用vue,在h5端和微信小程序端,通过编程实现访问后端的http://localhost:9000/mvc/upload的接口,其中图片服务器的路径为http://localhost:9000/image,实现图片上传的功能,请写出js api中的核心代码
时间: 2024-01-11 09:02:39 浏览: 29
请问您需要如何实现图片上传功能?常见的做法有两种:
1. 使用表单上传
2. 使用ajax上传
如果您采用第一种方式,可以使用以下代码:
在Vue文件中的Template标签中增加以下代码
```html
<form id="uploadForm" enctype="multipart/form-data">
<input type="file" id="file" name="file" />
<input type="button" value="上传" onclick="upload()" />
</form>
```
在Script标签中增加以下代码
```javascript
function upload() {
var formData = new FormData();
formData.append('file', $('#file')[0].files[0]);
$.ajax({
url: 'http://localhost:9000/mvc/upload',
type: 'POST',
data: formData,
dataType: 'json',
processData: false,
contentType: false,
success: function(data) {
console.log(data);
alert('上传成功!');
},
error: function(xhr, textStatus, errorThrown) {
console.log(xhr.responseText);
alert('上传失败!');
}
});
}
```
如果您选择使用ajax上传,可以使用以下代码:
```javascript
function upload() {
var formData = new FormData();
formData.append('file', $('#file')[0].files[0]);
$.ajax({
url: 'http://localhost:9000/mvc/upload',
type: 'POST',
data: formData,
dataType: 'json',
processData: false,
contentType: false,
success: function(data) {
console.log(data);
alert('上传成功!');
},
error: function(xhr, textStatus, errorThrown) {
console.log(xhr.responseText);
alert('上传失败!');
}
});
}
```
在以上代码中,需要注意几点:
- 在FormData中添加文件对象时,需要注意name值应该与后端接口中对应的参数名称一致,一般为“file”。
- processData和contentType设置为false,这样ajax会告诉服务器不要处理数据和设置请求头,以便正确处理文件二进制数据。
- success和error分别对应上传成功和上传失败的处理函数,可以根据需要自行修改。
相关推荐
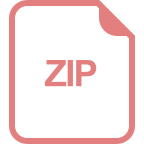
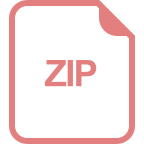
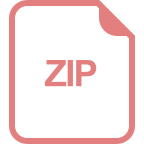














