from collections.abc import Iterable
时间: 2024-09-20 19:12:06 浏览: 29
`from collections.abc import Iterable` 这一行引入的是 Python 标准库 `collections` 模块中的抽象基类(ABCs),其中 `Iterable` 是一种特殊的 ABC,它定义了一个接口,用于标识那些可以迭代的对象,即可以逐个元素访问的数据结构。通过这个抽象概念,Python 可以确定哪些类型的对象支持迭代行为。
要理解 `Iterable`,你可以这样操作:
1. **判断对象是否可迭代**[^2]:
```python
l = [1, 2, 3, 4] # 列表是可迭代的
t = (1, 2, 3, 4) # 元组也是可迭代的
d = {1: 2, 3: 4} # 字典虽然不是序列,但其键值对可以通过 `.keys()` 或 `.values()` 方法迭代
s = {1, 2, 3, 4} # 集合同样可迭代
print(isinstance(l, Iterable)) # 输出:True
print(isinstance(t, Iterable)) # 输出:True
print(isinstance(d, Iterable)) # 输出:True
print(isinstance(s, Iterable)) # 输出:True
```
这表明列表、元组、字典和集合都是 `Iterable` 的实例。实际上,任何实现了 `__iter__` 和 `__next__` 方法的对象都可以被认为是可迭代的。
相关问题
from collections.abc import Container失败怎么办
如果在 Python 3.x 版本中使用 `from collections.abc import Container` 导入 `collections.abc` 模块中的 `Container` 抽象类时失败了,可能是因为你使用的 Python 版本过低,不支持 `collections.abc` 模块。
在 Python 3.2 之前的版本中,`collections.abc` 模块并不存在,取而代之的是 `collections` 模块中的 `MutableSequence`、`Hashable` 等类。如果你使用的是 Python 3.2 及以上的版本,但仍然出现了导入失败的情况,可以尝试更新你的 Python 版本或者升级你的 Anaconda 环境。
如果你使用的是 Python 2.x 版本,可以使用以下方式导入 `collections` 模块中的 `Iterable` 抽象类:
```python
from collections import Iterable
```
需要注意的是,如果你的 Python 版本过低,可能没有这些抽象基类,所以最好升级到较新的 Python 版本。
DeprecationWarning: Using or importing the ABCs from 'collections' instead of from 'collections.abc' is deprecated since Python 3.3, and in 3.10 it will stop working from collections import Iterable
这个警告是因为你在使用Python标准库`collections`中的`Iterable`类时,没有使用建议的导入方式。在Python 3.3之前,`collections`模块中的ABCs(抽象基类)是以普通的类形式存在的,因此我们可以直接从`collections`模块中导入ABCs,例如:
```
from collections import Iterable
class MyIterableClass(Iterable):
# some code here
```
然而,从Python 3.3开始,`collections`模块中的ABCs被移动到了`collections.abc`模块中,并且在Python 3.10中将停止在`collections`模块中提供ABCs的支持。因此,为了确保代码的可移植性和向后兼容性,建议使用以下方式导入ABCs:
```
from collections.abc import Iterable
class MyIterableClass(Iterable):
# some code here
```
这样可以避免在将来的Python版本中出现警告或错误。如果你想消除这个警告,只需将导入语句中的`collections`改为`collections.abc`即可。
阅读全文
相关推荐
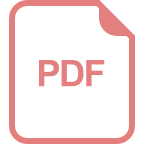
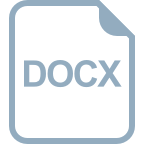
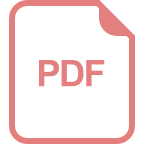


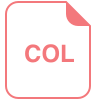










